Initial commit
195
addevent.php
Normal file
@ -0,0 +1,195 @@
|
||||
<?php
|
||||
session_start();
|
||||
if( $_SESSION['access'] != 1 ) {
|
||||
require( 'login.php' );
|
||||
} else {
|
||||
$uname=$_SESSION['uname'];
|
||||
?>
|
||||
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
|
||||
<html>
|
||||
<head>
|
||||
<link rel="stylesheet" type="text/css" href="css/sestyle.css">
|
||||
<link rel="shortcut icon" href="favicon.ico" />
|
||||
<script type="text/javascript" src="includes/jquery-2.1.3.min.js"></script>
|
||||
<link rel="stylesheet" href="//code.jquery.com/ui/1.11.4/themes/smoothness/jquery-ui.css">
|
||||
<link rel="stylesheet" type="text/css" href="includes/js/dtp/jquery.datetimepicker.css"/ >
|
||||
<script src="includes/js/dtp/jquery.js"></script>
|
||||
<script src="includes/js/dtp/jquery.datetimepicker.js"></script>
|
||||
<title>Add BMS Event</title>
|
||||
<script>
|
||||
$(function() {
|
||||
$('#start_date_time').datetimepicker();
|
||||
$('#end_date_time').datetimepicker();
|
||||
});
|
||||
</script>
|
||||
<script type="text/javascript">
|
||||
$(document).ready(function(){
|
||||
var queryString = $('select_form').serialize();
|
||||
$("select#utype").prop("disabled", true);
|
||||
$("select#unit").prop("disabled", true);
|
||||
$("select#alert").prop("disabled", true);
|
||||
$("select#datacenter").change(function(){
|
||||
$("select#utype").prop("disabled", true);
|
||||
/*$("select#utype").html("<option>wait...</option>");*/
|
||||
var dc_id = $("select#datacenter option:selected").val();
|
||||
$.post("includes/select_utype.php", function(data){
|
||||
$("select#utype").prop("disabled", false);
|
||||
$("select#utype").html(data);
|
||||
});
|
||||
});
|
||||
$("select#utype").change(function(){
|
||||
$("select#unit").prop("disabled", true);
|
||||
/*$("select#unit").html("<option>wait...</option>");*/
|
||||
var utype_id = $("select#utype option:selected").val();
|
||||
$.post("includes/select_unit.php", {utype_id:utype_id, dc_id:$('#datacenter').val()}, function(data){
|
||||
$("select#unit").prop("disabled", false);
|
||||
$("select#unit").html(data);
|
||||
});
|
||||
});
|
||||
$("select#unit").change(function(){
|
||||
$("select#alert").prop("disabled", true);
|
||||
var unit_id = $("select#unit option:selected").val();
|
||||
$.post("includes/select_alert.php", {utype_id:$('#utype').val()}, function(data){
|
||||
$("select#alert").prop("disabled", false);
|
||||
$("select#alert").html(data);
|
||||
});
|
||||
});
|
||||
$("form#select_form").submit(function(){
|
||||
var dc = $("select#datacenter option:selected").val();
|
||||
var utype = $("select#utype option:selected").val();
|
||||
if(cat>0 && type>0)
|
||||
{
|
||||
var result = $("select#utype option:selected").html();
|
||||
$("#result").html('your choice: '+result);
|
||||
}
|
||||
else
|
||||
{
|
||||
$("#result").html("you must choose a DC and Unit Type!");
|
||||
}
|
||||
return false;
|
||||
var alert = $("select#alert option:selected").val();
|
||||
if(cat>0 && type>0)
|
||||
{
|
||||
var result = $("select#alert option:selected").html();
|
||||
$("#result").html('your choice: '+result);
|
||||
}
|
||||
else
|
||||
{
|
||||
$("#result").html("you must choose a DC, Unit Type, and Alert!");
|
||||
}
|
||||
return false;
|
||||
var unit = $("select#unit option:selected").val();
|
||||
if(cat>0 && type>0)
|
||||
{
|
||||
var result = $("select#unit option:selected").html();
|
||||
$("#result").html('your choice: '+result);
|
||||
}
|
||||
else
|
||||
{
|
||||
$("#result").html("you must choose a DC, Unit Type, Alert, and Unit!");
|
||||
}
|
||||
return false;
|
||||
});
|
||||
});
|
||||
</script>
|
||||
</head>
|
||||
<body>
|
||||
<div class=header>
|
||||
<h1>Liquidweb BMS Events</h1>
|
||||
<?php
|
||||
if(isset($_SESSION['uname'])) {
|
||||
echo "Hello, ";
|
||||
print_r($_SESSION['uname']);
|
||||
}
|
||||
echo "<h2>Add an Event</h2>";
|
||||
include("includes/menu.php"); ?>
|
||||
</div>
|
||||
<?php include "includes/classes/select.class.php";?>
|
||||
<br />
|
||||
<form id="select_form" required method="post" action="includes/insert_event.php">
|
||||
<table align="center">
|
||||
<tr>
|
||||
<td>
|
||||
Choose a DC:
|
||||
</td>
|
||||
<td>
|
||||
<select name="datacenter" id="datacenter" required>
|
||||
<?php echo $opt->ShowDCs(); ?>
|
||||
</select>
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td>
|
||||
Choose a Unit Type:
|
||||
</td>
|
||||
<td>
|
||||
<select name="utype" id="utype" required>
|
||||
<?php echo $opt->ShowUnitType(); ?>
|
||||
</select>
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td>
|
||||
Choose a Unit:
|
||||
</td>
|
||||
<td>
|
||||
<select name="unit" id="unit" required>
|
||||
<?php echo $opt->ShowUnits(); ?>
|
||||
</select>
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td>
|
||||
Select the Alert:
|
||||
</td>
|
||||
<td>
|
||||
<select name="alert" id="alert" required>
|
||||
<?php echo $opt->ShowAlerts(); ?>
|
||||
</select>
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td>
|
||||
Start date and time:
|
||||
</td>
|
||||
<td>
|
||||
<input type="text" name="start_date_time" id="start_date_time" required />
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td>
|
||||
Issue Description:
|
||||
</td>
|
||||
<td>
|
||||
<input type="text" name="description" id="description" required />
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td>
|
||||
Currently Ongoing?
|
||||
</td>
|
||||
<td>
|
||||
<input type="checkbox" name="is_ongoing" value="1" id="is_ongoing" />
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td>
|
||||
End date and time:
|
||||
</td>
|
||||
<td>
|
||||
<input type="text" name="end_date_time" id="end_date_time" />
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td colspan="2" class="ui-helper-center">
|
||||
<input type="submit" value="Add Event" />
|
||||
</td>
|
||||
</tr>
|
||||
</table>
|
||||
You must press "Add Event" to record the issue!
|
||||
<input type="hidden" name="user" id="user" value="<?=$uname;?>"/>
|
||||
</form>
|
||||
<div id="result"></div>
|
||||
</body>
|
||||
</html>
|
||||
<?php } ?>
|
5
css/jquery.datetimeentry.css
Normal file
@ -0,0 +1,5 @@
|
||||
/* DateTimeEntry styles v2.0.0 */
|
||||
.datetimeEntry-control {
|
||||
vertical-align: middle;
|
||||
margin-left: 2px;
|
||||
}
|
5
css/jquery.timeentry.css
Normal file
@ -0,0 +1,5 @@
|
||||
/* TimeEntry styles v2.0.0 */
|
||||
.timeEntry-control {
|
||||
vertical-align: middle;
|
||||
margin-left: 2px;
|
||||
}
|
61
css/menu.css
Normal file
@ -0,0 +1,61 @@
|
||||
nav ul ul {
|
||||
display: none;
|
||||
}
|
||||
|
||||
nav ul li:hover > ul {
|
||||
display: block;
|
||||
}
|
||||
nav ul {
|
||||
color: #000000;
|
||||
background: #808080;
|
||||
background: linear-gradient(top, #808080 0%, #404040 100%);
|
||||
background: -moz-linear-gradient(top, #808080 0%, #404040 100%);
|
||||
background: -webkit-linear-gradient(top, #808080 0%,#404040 100%);
|
||||
box-shadow: 0px 0px 9px rgba(0,0,0,0.15);
|
||||
padding: 0 10px;
|
||||
border-radius: 10px;
|
||||
list-style: none;
|
||||
position: relative;
|
||||
display: inline-table;
|
||||
/* margin: 0.5em;*/
|
||||
}
|
||||
nav ul:after {
|
||||
content: ""; clear: both; display: block;
|
||||
}
|
||||
nav ul li {
|
||||
float: left;
|
||||
}
|
||||
nav ul li:hover {
|
||||
background: #505050;
|
||||
background: linear-gradient(top, #505050 0%, #585858 40%);
|
||||
background: -moz-linear-gradient(top, #505050 0%, #585858 40%);
|
||||
background: -webkit-linear-gradient(top, #505050 0%, #585858 40%);
|
||||
}
|
||||
nav ul li:hover a {
|
||||
color: #fff;
|
||||
}
|
||||
|
||||
nav ul li a {
|
||||
display: block; padding: 10px 10px;
|
||||
color: #FFFFFF; text-decoration: none;
|
||||
}
|
||||
nav ul ul {
|
||||
background: #505050; border-radius: 0px; padding: 0;
|
||||
position: absolute; top: 100%;
|
||||
}
|
||||
nav ul ul li {
|
||||
float: none;
|
||||
border-top: 1px solid #000000;
|
||||
border-bottom: 1px solid #000000;
|
||||
position: relative;
|
||||
}
|
||||
nav ul ul li a {
|
||||
padding: 5px 20px;
|
||||
color: #fff;
|
||||
}
|
||||
nav ul ul li a:hover {
|
||||
background: #585858;
|
||||
}
|
||||
nav ul ul ul {
|
||||
position: absolute; left: 100%; top:0;
|
||||
}
|
64
css/sestyle.css
Normal file
@ -0,0 +1,64 @@
|
||||
body {
|
||||
background-color: #E0E0E0;
|
||||
font-family: 'Source Sans Pro', 'Segoe UI', 'Droid Sans', Tahoma, Arial, sans-serif;
|
||||
text-align: center;
|
||||
}
|
||||
|
||||
.header {
|
||||
background-color: #313338;
|
||||
color: white;
|
||||
width: 100%;
|
||||
}
|
||||
.header h1 {
|
||||
color: #F8F8F8;
|
||||
text-align: center;
|
||||
font-family: 'Source Sans Pro', 'Segoe UI', 'Droid Sans', Tahoma, Arial, sans-serif;
|
||||
font-size: 1.75em;
|
||||
margin: 0.2em;
|
||||
}
|
||||
h3, h4, h5 {
|
||||
color: black;
|
||||
text-align: center;
|
||||
font-family: 'Source Sans Pro', 'Segoe UI', 'Droid Sans', Tahoma, Arial, sans-serif;
|
||||
}
|
||||
table, th, td {
|
||||
border: 1px solid black;
|
||||
text-align: left;
|
||||
border-collapse: collapse;
|
||||
padding: 3px;
|
||||
width: auto;
|
||||
}
|
||||
th {
|
||||
background-color: #3366CC;
|
||||
color: #F8F8F8;
|
||||
font-weight: normal;
|
||||
}
|
||||
tr:nth-child(odd) {
|
||||
background-color: white;
|
||||
}
|
||||
tr:nth-child(even) {
|
||||
background-color: lightgray;
|
||||
}
|
||||
td a, td a:link, td a:visited {
|
||||
font-weight:normal;
|
||||
color:black;
|
||||
background: none;
|
||||
width: auto;
|
||||
text-decoration: underline;
|
||||
text-transform: none;
|
||||
margin: 0px;
|
||||
padding: 3px;
|
||||
}
|
||||
th a, th a:link, th a:visited {
|
||||
font-weight:normal;
|
||||
color:white;
|
||||
background: none;
|
||||
width: auto;
|
||||
text-decoration: underline;
|
||||
text-transform: none;
|
||||
margin: 0px;
|
||||
padding: 3px;
|
||||
}
|
||||
.ui-helper-center {
|
||||
text-align:center;
|
||||
}
|
61
css/style.css
Normal file
@ -0,0 +1,61 @@
|
||||
body {
|
||||
background-color: #E0E0E0;
|
||||
font-family: 'Source Sans Pro', 'Segoe UI', 'Droid Sans', Tahoma, Arial, sans-serif;
|
||||
text-align: center;
|
||||
}
|
||||
|
||||
.header {
|
||||
background-color: #313338;
|
||||
color: white;
|
||||
width: 100%;
|
||||
}
|
||||
.header h1 {
|
||||
color: #F8F8F8;
|
||||
text-align: center;
|
||||
font-family: 'Source Sans Pro', 'Segoe UI', 'Droid Sans', Tahoma, Arial, sans-serif;
|
||||
font-size: 1.75em;
|
||||
margin: 0.2em;
|
||||
}
|
||||
h3, h4, h5 {
|
||||
color: black;
|
||||
text-align: center;
|
||||
font-family: 'Source Sans Pro', 'Segoe UI', 'Droid Sans', Tahoma, Arial, sans-serif;
|
||||
}
|
||||
table, th, td {
|
||||
border: 1px solid black;
|
||||
text-align: center;
|
||||
border-collapse: collapse;
|
||||
padding: 3px;
|
||||
width: auto;
|
||||
}
|
||||
th {
|
||||
background-color: #3366CC;
|
||||
color: #F8F8F8;
|
||||
font-weight: normal;
|
||||
}
|
||||
tr:nth-child(odd) {
|
||||
background-color: white;
|
||||
}
|
||||
tr:nth-child(even) {
|
||||
background-color: lightgray;
|
||||
}
|
||||
td a, td a:link, td a:visited {
|
||||
font-weight:normal;
|
||||
color:black;
|
||||
background: none;
|
||||
width: auto;
|
||||
text-decoration: underline;
|
||||
text-transform: none;
|
||||
margin: 0px;
|
||||
padding: 3px;
|
||||
}
|
||||
th a, th a:link, th a:visited {
|
||||
font-weight:normal;
|
||||
color:white;
|
||||
background: none;
|
||||
width: auto;
|
||||
text-decoration: underline;
|
||||
text-transform: none;
|
||||
margin: 0px;
|
||||
padding: 3px;
|
||||
}
|
172
editevent.php
Normal file
@ -0,0 +1,172 @@
|
||||
<?php
|
||||
session_start();
|
||||
if( $_SESSION['access'] != 1 ) {
|
||||
require( 'login.php' );
|
||||
} else {
|
||||
$uname=$_SESSION['uname'];
|
||||
?>
|
||||
|
||||
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
|
||||
<html>
|
||||
<head>
|
||||
<link rel="stylesheet" type="text/css" href="css/sestyle.css">
|
||||
<link rel="shortcut icon" href="favicon.ico" />
|
||||
<script type="text/javascript" src="includes/jquery-2.1.3.min.js"></script>
|
||||
<link rel="stylesheet" href="//code.jquery.com/ui/1.11.4/themes/smoothness/jquery-ui.css">
|
||||
<link rel="stylesheet" type="text/css" href="includes/js/dtp/jquery.datetimepicker.css"/ >
|
||||
<script src="includes/js/dtp/jquery.js"></script>
|
||||
<script src="includes/js/dtp/jquery.datetimepicker.js"></script>
|
||||
<title>Edit BMS Event</title>
|
||||
<script>
|
||||
$(function() {
|
||||
$('#start_date_time').datetimepicker();
|
||||
$('#end_date_time').datetimepicker();
|
||||
});
|
||||
</script>
|
||||
</head>
|
||||
<body>
|
||||
<div class=header>
|
||||
<h1>Liquidweb BMS Events</h1>
|
||||
<?php
|
||||
if(isset($_SESSION['uname'])) {
|
||||
echo "Hello, ";
|
||||
print_r($_SESSION['uname']);
|
||||
}
|
||||
echo "<h2>Edit Event</h2>";
|
||||
include("includes/menu.php"); ?>
|
||||
</div>
|
||||
<div class=body>
|
||||
<?php include "includes/db_config.php";
|
||||
//Get Event ID from URL
|
||||
if(isset($_GET['event_id']) && is_numeric($_GET['event_id'])) {
|
||||
$varEvent = $_GET['event_id'];
|
||||
} else {
|
||||
$varEvent = 0;
|
||||
}
|
||||
|
||||
$conn1 = new mysqli($servername, $username, $password, $db);
|
||||
if ($conn1->connect_error) {
|
||||
die ("Connection Failed: " . $conn1->connect_error);
|
||||
}
|
||||
$sql1 = "SELECT * FROM events WHERE event_id='".$varEvent."'";
|
||||
|
||||
$result1 = $conn1->query($sql1);
|
||||
if ($result1->num_rows >=1) {
|
||||
while($row1 = $result1->fetch_assoc()) {
|
||||
$unitid=$row1['unit_id'];
|
||||
$starttimedate=$row1['date_time_start'];
|
||||
$desc=$row1['description'];
|
||||
$ongoing=$row1['is_ongoing'];
|
||||
$endtimedate=$row1['date_time_end'];
|
||||
$alertid=$row1['alert_id'];
|
||||
$eventid=$row1['event_id'];
|
||||
}
|
||||
include "includes/classes/select2.class.php"; ?>
|
||||
<br />
|
||||
<form id="select_form" required method="post" action="includes/update_event.php">
|
||||
<table align=center>
|
||||
<tr>
|
||||
<td>
|
||||
Affected unit:
|
||||
</td>
|
||||
<td>
|
||||
<?php
|
||||
if ($conn1->connect_error) {
|
||||
die ("Connection Failed: " . $conn1->connect_error);
|
||||
}
|
||||
$sql2 = "SELECT unit_name FROM units WHERE unit_id='".$unitid."'";
|
||||
|
||||
$result2 = $conn1->query($sql2);
|
||||
if ($result2->num_rows >0) {
|
||||
while($row2 = $result2->fetch_assoc()) {
|
||||
$unitname=$row2['unit_name'];
|
||||
}
|
||||
} else {
|
||||
echo "Database Error";
|
||||
}
|
||||
echo $unitname;
|
||||
?>
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td>
|
||||
Alert:
|
||||
</td>
|
||||
<td>
|
||||
<?php
|
||||
if ($conn1->connect_error) {
|
||||
die ("Connection Failed: " . $conn1->connect_error);
|
||||
}
|
||||
$sql3 = "SELECT alert_name FROM alerts WHERE alert_id='".$alertid."'";
|
||||
|
||||
$result3 = $conn1->query($sql3);
|
||||
if ($result3->num_rows >0) {
|
||||
while($row3 = $result3->fetch_assoc()) {
|
||||
$alertname=$row3['alert_name'];
|
||||
}
|
||||
} else {
|
||||
echo "Database Error";
|
||||
}
|
||||
echo $alertname;
|
||||
$conn1->close();
|
||||
?>
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td>
|
||||
Start date and time:
|
||||
</td>
|
||||
<td>
|
||||
<?php
|
||||
echo $starttimedate;
|
||||
?>
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td>
|
||||
Issue Description:
|
||||
</td>
|
||||
<td>
|
||||
<input type="text" name="description" id="description" required value="<?=$desc;?>" />
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td>
|
||||
Currently Ongoing?
|
||||
</td>
|
||||
<td>
|
||||
<?php
|
||||
if ($ongoing == 1) $checked= ' checked="true"';
|
||||
|
||||
echo '<input type="checkbox" name="is_ongoing" id="is_ongoing" value="1"' . $checked .' />';
|
||||
?>
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td>
|
||||
End date and time:
|
||||
</td>
|
||||
<td>
|
||||
<input type="text" name="end_date_time" id="end_date_time" value="<?=$endtimedate;?>" />
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td colspan=2 class="ui-helper-center">
|
||||
<input type="submit" value="Submit" />
|
||||
</td>
|
||||
</tr>
|
||||
</table>
|
||||
You must press "Submit" to record the update!
|
||||
<input type="hidden" name="event" id="event" value="<?=$eventid;?>"/>
|
||||
<input type="hidden" name="user" id="user" value="<?=$uname;?>"/>
|
||||
</form>
|
||||
<?php
|
||||
}else{
|
||||
echo 'No entry found. <a href="javascript:history.back()">Go back</a>';
|
||||
}
|
||||
?>
|
||||
</div>
|
||||
<div id="result"></div>
|
||||
</body>
|
||||
</html>
|
||||
<?php } ?>
|
BIN
favicon.ico
Normal file
After Width: | Height: | Size: 1.1 KiB |
69
includes/classes/select.class.php
Normal file
@ -0,0 +1,69 @@
|
||||
<?php
|
||||
class SelectList
|
||||
{
|
||||
protected $conn;
|
||||
|
||||
public function __construct()
|
||||
{
|
||||
$this->DbConnect();
|
||||
}
|
||||
|
||||
protected function DbConnect()
|
||||
{
|
||||
include "/home/srv/html/bms/includes/db_config.php";
|
||||
$this->conn = mysql_connect($servername,$username,$password) OR die("Unable to connect to the database");
|
||||
mysql_select_db($db,$this->conn) OR die("can not select the database $db");
|
||||
return TRUE;
|
||||
}
|
||||
|
||||
public function ShowDCs()
|
||||
{
|
||||
$sql = "SELECT * FROM datacenters ORDER BY dc_name";
|
||||
$res = mysql_query($sql,$this->conn);
|
||||
$datacenter = '<option value="0">choose...</option>';
|
||||
while($row = mysql_fetch_array($res))
|
||||
{
|
||||
$datacenter .= '<option value="' . $row['dc_id'] . '">' . $row['dc_name'] . '</option>';
|
||||
}
|
||||
return $datacenter;
|
||||
}
|
||||
|
||||
public function ShowUnitType()
|
||||
{
|
||||
$sql = "SELECT * FROM unittypes ORDER BY utype_name";
|
||||
$res = mysql_query($sql,$this->conn);
|
||||
$unittype = '<option value="0">choose...</option>';
|
||||
while($row = mysql_fetch_array($res))
|
||||
{
|
||||
$unittype .= '<option value="' . $row['utype_id'] . '">' . $row['utype_name'] . '</option>';
|
||||
}
|
||||
return $unittype;
|
||||
}
|
||||
|
||||
public function ShowUnits()
|
||||
{
|
||||
$sql = "SELECT * FROM units WHERE utype_id=$_POST[utype_id] AND dc_id=$_POST[dc_id] ORDER BY unit_name";
|
||||
$res = mysql_query($sql,$this->conn);
|
||||
$unit = '<option value="0">choose...</option>';
|
||||
while($row = mysql_fetch_array($res))
|
||||
{
|
||||
$unit .= '<option value="' . $row['unit_id'] . '">' . $row['unit_name'] . '</option>';
|
||||
}
|
||||
return $unit;
|
||||
}
|
||||
|
||||
public function ShowAlerts()
|
||||
{
|
||||
$sql = "SELECT * FROM alerts WHERE utype_id=$_POST[utype_id] ORDER BY alert_name";
|
||||
$res = mysql_query($sql,$this->conn);
|
||||
$alert = '<option value="0">choose...</option>';
|
||||
while($row = mysql_fetch_array($res))
|
||||
{
|
||||
$alert .= '<option value="' . $row['alert_id'] . '">' . $row['alert_name'] . '</option>';
|
||||
}
|
||||
return $alert;
|
||||
}
|
||||
}
|
||||
|
||||
$opt = new SelectList();
|
||||
?>
|
69
includes/classes/select2.class.php
Normal file
@ -0,0 +1,69 @@
|
||||
<?php
|
||||
class SelectList
|
||||
{
|
||||
protected $conn;
|
||||
|
||||
public function __construct()
|
||||
{
|
||||
$this->DbConnect();
|
||||
}
|
||||
|
||||
protected function DbConnect()
|
||||
{
|
||||
include "/home/srv/html/bms/includes/db_config.php";
|
||||
$this->conn = mysql_connect($servername,$username,$password) OR die("Unable to connect to the database");
|
||||
mysql_select_db($db,$this->conn) OR die("can not select the database $db");
|
||||
return TRUE;
|
||||
}
|
||||
|
||||
public function ShowDCs()
|
||||
{
|
||||
$sql = "SELECT * FROM datacenters ORDER BY dc_name";
|
||||
$res = mysql_query($sql,$this->conn);
|
||||
$datacenter = '<option value="0">choose...</option>';
|
||||
while($row = mysql_fetch_array($res))
|
||||
{
|
||||
$datacenter .= '<option value="' . $row['dc_id'] . '">' . $row['dc_name'] . '</option>';
|
||||
}
|
||||
return $datacenter;
|
||||
}
|
||||
|
||||
public function ShowUnitType()
|
||||
{
|
||||
$sql = "SELECT * FROM unittypes order by utype_name";
|
||||
$res = mysql_query($sql,$this->conn);
|
||||
$unittype = '<option value="0">choose...</option>';
|
||||
while($row = mysql_fetch_array($res))
|
||||
{
|
||||
$unittype .= '<option value="' . $row['utype_id'] . '">' . $row['utype_name'] . '</option>';
|
||||
}
|
||||
return $unittype;
|
||||
}
|
||||
|
||||
public function ShowUnits()
|
||||
{
|
||||
$sql = "SELECT * FROM units ORDER BY unit_name";
|
||||
$res = mysql_query($sql,$this->conn);
|
||||
$unit = '<option value="0">choose...</option>';
|
||||
while($row = mysql_fetch_array($res))
|
||||
{
|
||||
$unit .= '<option value="' . $row['unit_id'] . '">' . $row['unit_name'] . '</option>';
|
||||
}
|
||||
return $unit;
|
||||
}
|
||||
|
||||
public function ShowAlerts()
|
||||
{
|
||||
$sql = "SELECT * FROM alerts ORDER BY alert_name";
|
||||
$res = mysql_query($sql,$this->conn);
|
||||
$alert = '<option value="0">choose...</option>';
|
||||
while($row = mysql_fetch_array($res))
|
||||
{
|
||||
$alert .= '<option value="' . $row['alert_id'] . '">' . $row['alert_name'] . '</option>';
|
||||
}
|
||||
return $alert;
|
||||
}
|
||||
}
|
||||
|
||||
$opt = new SelectList();
|
||||
?>
|
6
includes/db_config.php
Normal file
@ -0,0 +1,6 @@
|
||||
<?php
|
||||
$servername = 'localhost';
|
||||
$username = 'USERNAME';
|
||||
$password = 'PASSWORD';
|
||||
$db = 'DBNAME';
|
||||
?>
|
BIN
includes/images/spinnerBlue.png
Normal file
After Width: | Height: | Size: 439 B |
BIN
includes/images/spinnerBlueBig.png
Normal file
After Width: | Height: | Size: 974 B |
BIN
includes/images/spinnerDefault.png
Normal file
After Width: | Height: | Size: 374 B |
BIN
includes/images/spinnerDefaultBig.png
Normal file
After Width: | Height: | Size: 836 B |
BIN
includes/images/spinnerGem.png
Normal file
After Width: | Height: | Size: 325 B |
BIN
includes/images/spinnerGemBig.png
Normal file
After Width: | Height: | Size: 750 B |
BIN
includes/images/spinnerGreen.png
Normal file
After Width: | Height: | Size: 433 B |
BIN
includes/images/spinnerGreenBig.png
Normal file
After Width: | Height: | Size: 967 B |
BIN
includes/images/spinnerOrange.png
Normal file
After Width: | Height: | Size: 432 B |
BIN
includes/images/spinnerOrangeBig.png
Normal file
After Width: | Height: | Size: 966 B |
BIN
includes/images/spinnerSquare.png
Normal file
After Width: | Height: | Size: 1.3 KiB |
BIN
includes/images/spinnerSquareBig.png
Normal file
After Width: | Height: | Size: 1.3 KiB |
BIN
includes/images/spinnerText.png
Normal file
After Width: | Height: | Size: 424 B |
BIN
includes/images/spinnerTextBig.png
Normal file
After Width: | Height: | Size: 1.2 KiB |
BIN
includes/images/spinnerUpDown.png
Normal file
After Width: | Height: | Size: 718 B |
BIN
includes/images/spinnerUpDownBig.png
Normal file
After Width: | Height: | Size: 998 B |
25
includes/insert_event.php
Normal file
@ -0,0 +1,25 @@
|
||||
<?php
|
||||
include "db_config.php";
|
||||
$conn = mysqli_connect($servername, $username, $password, $db);
|
||||
|
||||
$unit = mysqli_real_escape_string($conn, $_POST['unit']);
|
||||
$start_date_time = mysqli_real_escape_string($conn, $_POST['start_date_time']);
|
||||
$description = mysqli_real_escape_string($conn, $_POST['description']);
|
||||
$is_ongoing = mysqli_real_escape_string($conn, $_POST['is_ongoing']);
|
||||
$end_date_time = mysqli_real_escape_string($conn, $_POST['end_date_time']);
|
||||
$alert = mysqli_real_escape_string($conn, $_POST['alert']);
|
||||
$user = mysqli_real_escape_string($conn, $_POST['user']);
|
||||
|
||||
//Insert event to events table
|
||||
$event = "INSERT INTO events (unit_id, date_time_start, description, is_ongoing, date_time_end, alert_id, user) VALUES ('$unit','$start_date_time', '$description', '$is_ongoing', '$end_date_time', '$alert', '$user')";
|
||||
|
||||
$result = mysqli_query($conn, $event);
|
||||
if($result){
|
||||
echo("Event added, redirecting...");
|
||||
sleep (2);
|
||||
header('Location: ../index.php');
|
||||
exit();
|
||||
} else{
|
||||
echo('Error! Please <a href="javascript:history.back()">go back</a> and try again');
|
||||
}
|
||||
?>
|
4
includes/jquery-2.1.3.min.js
vendored
Normal file
36
includes/js/.jscsrc
Normal file
@ -0,0 +1,36 @@
|
||||
{
|
||||
"disallowEmptyBlocks": true,
|
||||
"disallowKeywords": ["with"],
|
||||
"disallowMixedSpacesAndTabs": true,
|
||||
"disallowMultipleLineStrings": true,
|
||||
"disallowMultipleVarDecl": true,
|
||||
"disallowQuotedKeysInObjects": "allButReserved",
|
||||
"disallowSpaceAfterPrefixUnaryOperators": ["++", "--", "+", "-", "~", "!"],
|
||||
"disallowSpaceBeforeBinaryOperators": [","],
|
||||
"disallowSpaceBeforePostfixUnaryOperators": ["++", "--"],
|
||||
"disallowSpacesInNamedFunctionExpression": { "beforeOpeningRoundBrace": true },
|
||||
"disallowSpacesInsideArrayBrackets": true,
|
||||
"disallowSpacesInsideParentheses": true,
|
||||
"disallowTrailingComma": true,
|
||||
"disallowTrailingWhitespace": true,
|
||||
"requireCamelCaseOrUpperCaseIdentifiers": true,
|
||||
"requireCapitalizedConstructors": true,
|
||||
"requireCommaBeforeLineBreak": true,
|
||||
"requireDotNotation": true,
|
||||
"requireLineFeedAtFileEnd": true,
|
||||
"requireSpaceAfterBinaryOperators": ["+", "-", "/", "*", "=", "==", "===", "!=", "!==", ">", "<", ">=", "<="],
|
||||
"requireSpaceAfterKeywords": ["if", "else", "for", "while", "do", "switch", "return", "try", "catch"],
|
||||
"requireSpaceAfterLineComment": true,
|
||||
"requireSpaceBeforeBinaryOperators": ["+", "-", "/", "*", "=", "==", "===", "!=", "!==", ">", "<", ">=", "<="],
|
||||
"requireSpaceBetweenArguments": true,
|
||||
"requireSpacesInAnonymousFunctionExpression": { "beforeOpeningCurlyBrace": true, "beforeOpeningRoundBrace": true },
|
||||
"requireSpacesInConditionalExpression": true,
|
||||
"requireSpacesInForStatement": true,
|
||||
"requireSpacesInFunctionDeclaration": { "beforeOpeningCurlyBrace": true },
|
||||
"requireSpacesInFunctionExpression": { "beforeOpeningCurlyBrace": true },
|
||||
"requireSpacesInNamedFunctionExpression": { "beforeOpeningCurlyBrace": true },
|
||||
"requireSpacesInsideObjectBrackets": "allButNested",
|
||||
"validateIndentation": 2,
|
||||
"validateLineBreaks": "LF",
|
||||
"validateQuoteMarks": "'"
|
||||
}
|
15
includes/js/.jshintrc
Normal file
@ -0,0 +1,15 @@
|
||||
{
|
||||
"asi" : true,
|
||||
"browser" : true,
|
||||
"eqeqeq" : false,
|
||||
"eqnull" : true,
|
||||
"es3" : true,
|
||||
"expr" : true,
|
||||
"jquery" : true,
|
||||
"latedef" : true,
|
||||
"laxbreak" : true,
|
||||
"nonbsp" : true,
|
||||
"strict" : true,
|
||||
"undef" : true,
|
||||
"unused" : true
|
||||
}
|
2
includes/js/dtp/.gitignore
vendored
Normal file
@ -0,0 +1,2 @@
|
||||
$ cat .gitignore
|
||||
parse.php
|
19
includes/js/dtp/MIT-LICENSE.txt
Normal file
@ -0,0 +1,19 @@
|
||||
Copyright (c) 2013 http://xdsoft.net
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in
|
||||
all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
||||
THE SOFTWARE.
|
20
includes/js/dtp/README.md
Normal file
@ -0,0 +1,20 @@
|
||||
datetimepicker
|
||||
==============
|
||||
[Documentation][doc]
|
||||
|
||||
|
||||
jQuery Plugin Date and Time Picker
|
||||
|
||||
DateTimePicker
|
||||
|
||||
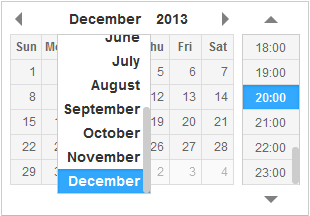
|
||||
|
||||
DatePicker
|
||||
|
||||

|
||||
|
||||
TimePicker
|
||||
|
||||

|
||||
|
||||
[doc]: http://xdsoft.net/jqplugins/datetimepicker/
|
50
includes/js/dtp/bower.json
Normal file
@ -0,0 +1,50 @@
|
||||
{
|
||||
"name":"datetimepicker",
|
||||
"version":"2.4.1",
|
||||
"main": [
|
||||
"jquery.datetimepicker.js",
|
||||
"jquery.datetimepicker.css"
|
||||
],
|
||||
"ignore": [
|
||||
"**/screen",
|
||||
"**/datetimepicker.jquery.json",
|
||||
"**/*.png",
|
||||
"**/*.txt",
|
||||
"**/*.md",
|
||||
"**/*.html",
|
||||
"**/*.tpl",
|
||||
"**/jquery.js"
|
||||
],
|
||||
"keywords": [
|
||||
"calendar",
|
||||
"date",
|
||||
"time",
|
||||
"form",
|
||||
"datetime",
|
||||
"datepicker",
|
||||
"timepicker",
|
||||
"datetimepicker",
|
||||
"validation",
|
||||
"ui",
|
||||
"scroller",
|
||||
"picker",
|
||||
"i18n",
|
||||
"input",
|
||||
"jquery",
|
||||
"touch"
|
||||
],
|
||||
"dependencies": {
|
||||
"jquery": ">= 1.7.2"
|
||||
},
|
||||
"authors": [
|
||||
{
|
||||
"name": "Chupurnov Valeriy",
|
||||
"email": "chupurnov@gmail.com",
|
||||
"homepage": "http://xdsoft.net/contacts.html"
|
||||
}
|
||||
],
|
||||
"homepage":"http://xdsoft.net/jqplugins/datetimepicker/",
|
||||
"repository": {
|
||||
"type": "git", "url": "git://github.com:xdan/datetimepicker.git"
|
||||
}
|
||||
}
|
47
includes/js/dtp/datetimepicker.jquery.json
Normal file
@ -0,0 +1,47 @@
|
||||
{
|
||||
"name": "datetimepicker",
|
||||
"version": "2.4.1",
|
||||
"title": "jQuery Date and Time picker",
|
||||
"description": "jQuery plugin for date, time, or datetime manipulation in form",
|
||||
"keywords": [
|
||||
"calendar",
|
||||
"date",
|
||||
"time",
|
||||
"form",
|
||||
"datetime",
|
||||
"datepicker",
|
||||
"timepicker",
|
||||
"datetimepicker",
|
||||
"validation",
|
||||
"ui",
|
||||
"scroller",
|
||||
"picker",
|
||||
"i18n",
|
||||
"input",
|
||||
"jquery",
|
||||
"touch"
|
||||
],
|
||||
"author": {
|
||||
"name": "Chupurnov Valeriy",
|
||||
"email": "chupurnov@gmail.com",
|
||||
"url": "http://xdsoft.net/contacts.html"
|
||||
},
|
||||
"maintainers": [{
|
||||
"name": "Chupurnov Valeriy",
|
||||
"email": "chupurnov@gmail.com",
|
||||
"url": "http://xdsoft.net"
|
||||
}],
|
||||
"licenses": [
|
||||
{
|
||||
"type": "MIT",
|
||||
"url": "https://github.com/xdan/datetimepicker/blob/master/MIT-LICENSE.txt"
|
||||
}
|
||||
],
|
||||
"bugs": "https://github.com/xdan/datetimepicker/issues",
|
||||
"homepage": "http://xdsoft.net/jqplugins/datetimepicker/",
|
||||
"docs": "http://xdsoft.net/jqplugins/datetimepicker/",
|
||||
"download": "https://github.com/xdan/datetimepicker/archive/master.zip",
|
||||
"dependencies": {
|
||||
"jquery": ">=1.7"
|
||||
}
|
||||
}
|
190
includes/js/dtp/index.html
Normal file
@ -0,0 +1,190 @@
|
||||
<!DOCTYPE html>
|
||||
<html lang="en">
|
||||
<head>
|
||||
<meta http-equiv="content-type" content="text/html; charset=utf-8"/>
|
||||
<link rel="stylesheet" type="text/css" href="./jquery.datetimepicker.css"/>
|
||||
<style type="text/css">
|
||||
|
||||
.custom-date-style {
|
||||
background-color: red !important;
|
||||
}
|
||||
|
||||
</style>
|
||||
</head>
|
||||
<body>
|
||||
|
||||
<p><a href="http://xdsoft.net/jqplugins/datetimepicker/">Homepage</a></p>
|
||||
<h3>DateTimePicker</h3>
|
||||
<input type="text" value="" id="datetimepicker"/><br><br>
|
||||
<h3>DateTimePickers selected by class</h3>
|
||||
<input type="text" class="some_class" value="" id="some_class_1"/>
|
||||
<input type="text" class="some_class" value="" id="some_class_2"/>
|
||||
<h3>Mask DateTimePicker</h3>
|
||||
<input type="text" value="" id="datetimepicker_mask"/><br><br>
|
||||
<h3>TimePicker</h3>
|
||||
<input type="text" id="datetimepicker1"/><br><br>
|
||||
<h3>DatePicker</h3>
|
||||
<input type="text" id="datetimepicker2"/><br><br>
|
||||
<h3>Inline DateTimePicker</h3>
|
||||
<!--<div id="console" style="background-color:#fff;color:red">sdfdsfsdf</div>-->
|
||||
<input type="text" id="datetimepicker3"/><input type="button" onclick="$('#datetimepicker3').datetimepicker({value:'2011/12/11 12:00'})" value="set inline value 2011/12/11 12:00"/><br><br>
|
||||
<h3>Button Trigger</h3>
|
||||
<input type="text" value="2013/12/03 18:00" id="datetimepicker4"/><input id="open" type="button" value="open"/><input id="close" type="button" value="close"/><input id="reset" type="button" value="reset"/>
|
||||
<h3>TimePicker allows time</h3>
|
||||
<input type="text" id="datetimepicker5"/><br><br>
|
||||
<h3>Destroy DateTimePicker</h3>
|
||||
<input type="text" id="datetimepicker6"/><input id="destroy" type="button" value="destroy"/>
|
||||
<h3>Set options runtime DateTimePicker</h3>
|
||||
<input type="text" id="datetimepicker7"/>
|
||||
<p>If select day is Saturday, the minimum set 11:00, otherwise 8:00</p>
|
||||
<h3>onGenerate</h3>
|
||||
<input type="text" id="datetimepicker8"/>
|
||||
<h3>disable all weekend</h3>
|
||||
<input type="text" id="datetimepicker9"/>
|
||||
<h3>Default date and time </h3>
|
||||
<input type="text" id="default_datetimepicker"/>
|
||||
<h3>Show inline</h3>
|
||||
<a href="javascript:void(0)" onclick="var si = document.getElementById('show_inline').style; si.display = (si.display=='none')?'block':'none';return false; ">Show/Hide</a>
|
||||
<div id="show_inline" style="display:none">
|
||||
<input type="text" id="datetimepicker10"/>
|
||||
</div>
|
||||
<h3>Disable Specific Dates</h3>
|
||||
<p>Disable the dates 2 days from now.</p>
|
||||
<input type="text" id="datetimepicker11"/>
|
||||
<h3>Custom Date Styling</h3>
|
||||
<p>Make the background of the date 2 days from now bright red.</p>
|
||||
<input type="text" id="datetimepicker12"/>
|
||||
<h3>Dark theme</h3>
|
||||
<p>thank for this <a href="https://github.com/lampslave">https://github.com/lampslave</a></p>
|
||||
<input type="text" id="datetimepicker_dark"/>
|
||||
</body>
|
||||
<script src="./jquery.js"></script>
|
||||
<script src="./jquery.datetimepicker.js"></script>
|
||||
<script>/*
|
||||
window.onerror = function(errorMsg) {
|
||||
$('#console').html($('#console').html()+'<br>'+errorMsg)
|
||||
}*/
|
||||
$('#datetimepicker').datetimepicker({
|
||||
dayOfWeekStart : 1,
|
||||
lang:'en',
|
||||
disabledDates:['1986/01/08','1986/01/09','1986/01/10'],
|
||||
startDate: '1986/01/05'
|
||||
});
|
||||
$('#datetimepicker').datetimepicker({value:'2015/04/15 05:03',step:10});
|
||||
|
||||
$('.some_class').datetimepicker();
|
||||
|
||||
$('#default_datetimepicker').datetimepicker({
|
||||
formatTime:'H:i',
|
||||
formatDate:'d.m.Y',
|
||||
defaultDate:'8.12.1986', // it's my birthday
|
||||
defaultTime:'10:00',
|
||||
timepickerScrollbar:false
|
||||
});
|
||||
|
||||
$('#datetimepicker10').datetimepicker({
|
||||
step:5,
|
||||
inline:true
|
||||
});
|
||||
$('#datetimepicker_mask').datetimepicker({
|
||||
mask:'9999/19/39 29:59'
|
||||
});
|
||||
|
||||
$('#datetimepicker1').datetimepicker({
|
||||
datepicker:false,
|
||||
format:'H:i',
|
||||
step:5
|
||||
});
|
||||
$('#datetimepicker2').datetimepicker({
|
||||
yearOffset:222,
|
||||
lang:'ch',
|
||||
timepicker:false,
|
||||
format:'d/m/Y',
|
||||
formatDate:'Y/m/d',
|
||||
minDate:'-1970/01/02', // yesterday is minimum date
|
||||
maxDate:'+1970/01/02' // and tommorow is maximum date calendar
|
||||
});
|
||||
$('#datetimepicker3').datetimepicker({
|
||||
inline:true
|
||||
});
|
||||
$('#datetimepicker4').datetimepicker();
|
||||
$('#open').click(function(){
|
||||
$('#datetimepicker4').datetimepicker('show');
|
||||
});
|
||||
$('#close').click(function(){
|
||||
$('#datetimepicker4').datetimepicker('hide');
|
||||
});
|
||||
$('#reset').click(function(){
|
||||
$('#datetimepicker4').datetimepicker('reset');
|
||||
});
|
||||
$('#datetimepicker5').datetimepicker({
|
||||
datepicker:false,
|
||||
allowTimes:['12:00','13:00','15:00','17:00','17:05','17:20','19:00','20:00'],
|
||||
step:5
|
||||
});
|
||||
$('#datetimepicker6').datetimepicker();
|
||||
$('#destroy').click(function(){
|
||||
if( $('#datetimepicker6').data('xdsoft_datetimepicker') ){
|
||||
$('#datetimepicker6').datetimepicker('destroy');
|
||||
this.value = 'create';
|
||||
}else{
|
||||
$('#datetimepicker6').datetimepicker();
|
||||
this.value = 'destroy';
|
||||
}
|
||||
});
|
||||
var logic = function( currentDateTime ){
|
||||
if( currentDateTime.getDay()==6 ){
|
||||
this.setOptions({
|
||||
minTime:'11:00'
|
||||
});
|
||||
}else
|
||||
this.setOptions({
|
||||
minTime:'8:00'
|
||||
});
|
||||
};
|
||||
$('#datetimepicker7').datetimepicker({
|
||||
onChangeDateTime:logic,
|
||||
onShow:logic
|
||||
});
|
||||
$('#datetimepicker8').datetimepicker({
|
||||
onGenerate:function( ct ){
|
||||
$(this).find('.xdsoft_date')
|
||||
.toggleClass('xdsoft_disabled');
|
||||
},
|
||||
minDate:'-1970/01/2',
|
||||
maxDate:'+1970/01/2',
|
||||
timepicker:false
|
||||
});
|
||||
$('#datetimepicker9').datetimepicker({
|
||||
onGenerate:function( ct ){
|
||||
$(this).find('.xdsoft_date.xdsoft_weekend')
|
||||
.addClass('xdsoft_disabled');
|
||||
},
|
||||
weekends:['01.01.2014','02.01.2014','03.01.2014','04.01.2014','05.01.2014','06.01.2014'],
|
||||
timepicker:false
|
||||
});
|
||||
var dateToDisable = new Date();
|
||||
dateToDisable.setDate(dateToDisable.getDate() + 2);
|
||||
$('#datetimepicker11').datetimepicker({
|
||||
beforeShowDay: function(date) {
|
||||
if (date.getMonth() == dateToDisable.getMonth() && date.getDate() == dateToDisable.getDate()) {
|
||||
return [false, ""]
|
||||
}
|
||||
|
||||
return [true, ""];
|
||||
}
|
||||
});
|
||||
$('#datetimepicker12').datetimepicker({
|
||||
beforeShowDay: function(date) {
|
||||
if (date.getMonth() == dateToDisable.getMonth() && date.getDate() == dateToDisable.getDate()) {
|
||||
return [true, "custom-date-style"];
|
||||
}
|
||||
|
||||
return [true, ""];
|
||||
}
|
||||
});
|
||||
$('#datetimepicker_dark').datetimepicker({theme:'dark'})
|
||||
|
||||
|
||||
</script>
|
||||
</html>
|
523
includes/js/dtp/jquery.datetimepicker.css
Normal file
@ -0,0 +1,523 @@
|
||||
.xdsoft_datetimepicker {
|
||||
box-shadow: 0 5px 15px -5px rgba(0, 0, 0, 0.506);
|
||||
background: #fff;
|
||||
border-bottom: 1px solid #bbb;
|
||||
border-left: 1px solid #ccc;
|
||||
border-right: 1px solid #ccc;
|
||||
border-top: 1px solid #ccc;
|
||||
color: #333;
|
||||
font-family: "Helvetica Neue", Helvetica, Arial, sans-serif;
|
||||
padding: 8px;
|
||||
padding-left: 0;
|
||||
padding-top: 2px;
|
||||
position: absolute;
|
||||
z-index: 9999;
|
||||
-moz-box-sizing: border-box;
|
||||
box-sizing: border-box;
|
||||
display: none;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker iframe {
|
||||
position: absolute;
|
||||
left: 0;
|
||||
top: 0;
|
||||
width: 75px;
|
||||
height: 210px;
|
||||
background: transparent;
|
||||
border: none;
|
||||
}
|
||||
|
||||
/*For IE8 or lower*/
|
||||
.xdsoft_datetimepicker button {
|
||||
border: none !important;
|
||||
}
|
||||
|
||||
.xdsoft_noselect {
|
||||
-webkit-touch-callout: none;
|
||||
-webkit-user-select: none;
|
||||
-khtml-user-select: none;
|
||||
-moz-user-select: none;
|
||||
-ms-user-select: none;
|
||||
-o-user-select: none;
|
||||
user-select: none;
|
||||
}
|
||||
|
||||
.xdsoft_noselect::selection { background: transparent }
|
||||
.xdsoft_noselect::-moz-selection { background: transparent }
|
||||
|
||||
.xdsoft_datetimepicker.xdsoft_inline {
|
||||
display: inline-block;
|
||||
position: static;
|
||||
box-shadow: none;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker * {
|
||||
-moz-box-sizing: border-box;
|
||||
box-sizing: border-box;
|
||||
padding: 0;
|
||||
margin: 0;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_datepicker, .xdsoft_datetimepicker .xdsoft_timepicker {
|
||||
display: none;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_datepicker.active, .xdsoft_datetimepicker .xdsoft_timepicker.active {
|
||||
display: block;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_datepicker {
|
||||
width: 224px;
|
||||
float: left;
|
||||
margin-left: 8px;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker.xdsoft_showweeks .xdsoft_datepicker {
|
||||
width: 256px;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_timepicker {
|
||||
width: 58px;
|
||||
float: left;
|
||||
text-align: center;
|
||||
margin-left: 8px;
|
||||
margin-top: 0;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_datepicker.active+.xdsoft_timepicker {
|
||||
margin-top: 8px;
|
||||
margin-bottom: 3px
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_mounthpicker {
|
||||
position: relative;
|
||||
text-align: center;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_label i,
|
||||
.xdsoft_datetimepicker .xdsoft_prev,
|
||||
.xdsoft_datetimepicker .xdsoft_next,
|
||||
.xdsoft_datetimepicker .xdsoft_today_button {
|
||||
background-image: url(data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAGQAAAAeCAYAAADaW7vzAAAAGXRFWHRTb2Z0d2FyZQBBZG9iZSBJbWFnZVJlYWR5ccllPAAAAyJpVFh0WE1MOmNvbS5hZG9iZS54bXAAAAAAADw/eHBhY2tldCBiZWdpbj0i77u/IiBpZD0iVzVNME1wQ2VoaUh6cmVTek5UY3prYzlkIj8+IDx4OnhtcG1ldGEgeG1sbnM6eD0iYWRvYmU6bnM6bWV0YS8iIHg6eG1wdGs9IkFkb2JlIFhNUCBDb3JlIDUuMy1jMDExIDY2LjE0NTY2MSwgMjAxMi8wMi8wNi0xNDo1NjoyNyAgICAgICAgIj4gPHJkZjpSREYgeG1sbnM6cmRmPSJodHRwOi8vd3d3LnczLm9yZy8xOTk5LzAyLzIyLXJkZi1zeW50YXgtbnMjIj4gPHJkZjpEZXNjcmlwdGlvbiByZGY6YWJvdXQ9IiIgeG1sbnM6eG1wPSJodHRwOi8vbnMuYWRvYmUuY29tL3hhcC8xLjAvIiB4bWxuczp4bXBNTT0iaHR0cDovL25zLmFkb2JlLmNvbS94YXAvMS4wL21tLyIgeG1sbnM6c3RSZWY9Imh0dHA6Ly9ucy5hZG9iZS5jb20veGFwLzEuMC9zVHlwZS9SZXNvdXJjZVJlZiMiIHhtcDpDcmVhdG9yVG9vbD0iQWRvYmUgUGhvdG9zaG9wIENTNiAoV2luZG93cykiIHhtcE1NOkluc3RhbmNlSUQ9InhtcC5paWQ6Q0NBRjI1NjM0M0UwMTFFNDk4NkFGMzJFQkQzQjEwRUIiIHhtcE1NOkRvY3VtZW50SUQ9InhtcC5kaWQ6Q0NBRjI1NjQ0M0UwMTFFNDk4NkFGMzJFQkQzQjEwRUIiPiA8eG1wTU06RGVyaXZlZEZyb20gc3RSZWY6aW5zdGFuY2VJRD0ieG1wLmlpZDpDQ0FGMjU2MTQzRTAxMUU0OTg2QUYzMkVCRDNCMTBFQiIgc3RSZWY6ZG9jdW1lbnRJRD0ieG1wLmRpZDpDQ0FGMjU2MjQzRTAxMUU0OTg2QUYzMkVCRDNCMTBFQiIvPiA8L3JkZjpEZXNjcmlwdGlvbj4gPC9yZGY6UkRGPiA8L3g6eG1wbWV0YT4gPD94cGFja2V0IGVuZD0iciI/PoNEP54AAAIOSURBVHja7Jq9TsMwEMcxrZD4WpBYeKUCe+kTMCACHZh4BFfHO/AAIHZGFhYkBBsSEqxsLCAgXKhbXYOTxh9pfJVP+qutnZ5s/5Lz2Y5I03QhWji2GIcgAokWgfCxNvcOCCGKqiSqhUp0laHOne05vdEyGMfkdxJDVjgwDlEQgYQBgx+ULJaWSXXS6r/ER5FBVR8VfGftTKcITNs+a1XpcFoExREIDF14AVIFxgQUS+h520cdud6wNkC0UBw6BCO/HoCYwBhD8QCkQ/x1mwDyD4plh4D6DDV0TAGyo4HcawLIBBSLDkHeH0Mg2yVP3l4TQMZQDDsEOl/MgHQqhMNuE0D+oBh0CIr8MAKyazBH9WyBuKxDWgbXfjNf32TZ1KWm/Ap1oSk/R53UtQ5xTh3LUlMmT8gt6g51Q9p+SobxgJQ/qmsfZhWywGFSl0yBjCLJCMgXail3b7+rumdVJ2YRss4cN+r6qAHDkPWjPjdJCF4n9RmAD/V9A/Wp4NQassDjwlB6XBiCxcJQWmZZb8THFilfy/lfrTvLghq2TqTHrRMTKNJ0sIhdo15RT+RpyWwFdY96UZ/LdQKBGjcXpcc1AlSFEfLmouD+1knuxBDUVrvOBmoOC/rEcN7OQxKVeJTCiAdUzUJhA2Oez9QTkp72OTVcxDcXY8iKNkxGAJXmJCOQwOa6dhyXsOa6XwEGAKdeb5ET3rQdAAAAAElFTkSuQmCC);
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_label i {
|
||||
opacity: 0.5;
|
||||
background-position: -92px -19px;
|
||||
display: inline-block;
|
||||
width: 9px;
|
||||
height: 20px;
|
||||
vertical-align: middle;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_prev {
|
||||
float: left;
|
||||
background-position: -20px 0;
|
||||
}
|
||||
.xdsoft_datetimepicker .xdsoft_today_button {
|
||||
float: left;
|
||||
background-position: -70px 0;
|
||||
margin-left: 5px;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_next {
|
||||
float: right;
|
||||
background-position: 0 0;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_next,
|
||||
.xdsoft_datetimepicker .xdsoft_prev ,
|
||||
.xdsoft_datetimepicker .xdsoft_today_button {
|
||||
background-color: transparent;
|
||||
background-repeat: no-repeat;
|
||||
border: 0 none;
|
||||
cursor: pointer;
|
||||
display: block;
|
||||
height: 30px;
|
||||
opacity: 0.5;
|
||||
-ms-filter: "progid:DXImageTransform.Microsoft.Alpha(Opacity=50)";
|
||||
outline: medium none;
|
||||
overflow: hidden;
|
||||
padding: 0;
|
||||
position: relative;
|
||||
text-indent: 100%;
|
||||
white-space: nowrap;
|
||||
width: 20px;
|
||||
min-width: 0;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_timepicker .xdsoft_prev,
|
||||
.xdsoft_datetimepicker .xdsoft_timepicker .xdsoft_next {
|
||||
float: none;
|
||||
background-position: -40px -15px;
|
||||
height: 15px;
|
||||
width: 30px;
|
||||
display: block;
|
||||
margin-left: 14px;
|
||||
margin-top: 7px;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_timepicker .xdsoft_prev {
|
||||
background-position: -40px 0;
|
||||
margin-bottom: 7px;
|
||||
margin-top: 0;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_timepicker .xdsoft_time_box {
|
||||
height: 151px;
|
||||
overflow: hidden;
|
||||
border-bottom: 1px solid #ddd;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_timepicker .xdsoft_time_box >div >div {
|
||||
background: #f5f5f5;
|
||||
border-top: 1px solid #ddd;
|
||||
color: #666;
|
||||
font-size: 12px;
|
||||
text-align: center;
|
||||
border-collapse: collapse;
|
||||
cursor: pointer;
|
||||
border-bottom-width: 0;
|
||||
height: 25px;
|
||||
line-height: 25px;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_timepicker .xdsoft_time_box >div > div:first-child {
|
||||
border-top-width: 0;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_today_button:hover,
|
||||
.xdsoft_datetimepicker .xdsoft_next:hover,
|
||||
.xdsoft_datetimepicker .xdsoft_prev:hover {
|
||||
opacity: 1;
|
||||
-ms-filter: "progid:DXImageTransform.Microsoft.Alpha(Opacity=100)";
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_label {
|
||||
display: inline;
|
||||
position: relative;
|
||||
z-index: 9999;
|
||||
margin: 0;
|
||||
padding: 5px 3px;
|
||||
font-size: 14px;
|
||||
line-height: 20px;
|
||||
font-weight: bold;
|
||||
background-color: #fff;
|
||||
float: left;
|
||||
width: 182px;
|
||||
text-align: center;
|
||||
cursor: pointer;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_label:hover>span {
|
||||
text-decoration: underline;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_label:hover i {
|
||||
opacity: 1.0;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_label > .xdsoft_select {
|
||||
border: 1px solid #ccc;
|
||||
position: absolute;
|
||||
right: 0;
|
||||
top: 30px;
|
||||
z-index: 101;
|
||||
display: none;
|
||||
background: #fff;
|
||||
max-height: 160px;
|
||||
overflow-y: hidden;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_label > .xdsoft_select.xdsoft_monthselect{ right: -7px }
|
||||
.xdsoft_datetimepicker .xdsoft_label > .xdsoft_select.xdsoft_yearselect{ right: 2px }
|
||||
.xdsoft_datetimepicker .xdsoft_label > .xdsoft_select > div > .xdsoft_option:hover {
|
||||
color: #fff;
|
||||
background: #ff8000;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_label > .xdsoft_select > div > .xdsoft_option {
|
||||
padding: 2px 10px 2px 5px;
|
||||
text-decoration: none !important;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_label > .xdsoft_select > div > .xdsoft_option.xdsoft_current {
|
||||
background: #33aaff;
|
||||
box-shadow: #178fe5 0 1px 3px 0 inset;
|
||||
color: #fff;
|
||||
font-weight: 700;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_month {
|
||||
width: 100px;
|
||||
text-align: right;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_calendar {
|
||||
clear: both;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_year{
|
||||
width: 48px;
|
||||
margin-left: 5px;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_calendar table {
|
||||
border-collapse: collapse;
|
||||
width: 100%;
|
||||
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_calendar td > div {
|
||||
padding-right: 5px;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_calendar th {
|
||||
height: 25px;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_calendar td,.xdsoft_datetimepicker .xdsoft_calendar th {
|
||||
width: 14.2857142%;
|
||||
background: #f5f5f5;
|
||||
border: 1px solid #ddd;
|
||||
color: #666;
|
||||
font-size: 12px;
|
||||
text-align: right;
|
||||
vertical-align: middle;
|
||||
padding: 0;
|
||||
border-collapse: collapse;
|
||||
cursor: pointer;
|
||||
height: 25px;
|
||||
}
|
||||
.xdsoft_datetimepicker.xdsoft_showweeks .xdsoft_calendar td,.xdsoft_datetimepicker.xdsoft_showweeks .xdsoft_calendar th {
|
||||
width: 12.5%;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_calendar th {
|
||||
background: #f1f1f1;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_calendar td.xdsoft_today {
|
||||
color: #33aaff;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_calendar td.xdsoft_default,
|
||||
.xdsoft_datetimepicker .xdsoft_calendar td.xdsoft_current,
|
||||
.xdsoft_datetimepicker .xdsoft_timepicker .xdsoft_time_box >div >div.xdsoft_current {
|
||||
background: #33aaff;
|
||||
box-shadow: #178fe5 0 1px 3px 0 inset;
|
||||
color: #fff;
|
||||
font-weight: 700;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_calendar td.xdsoft_other_month,
|
||||
.xdsoft_datetimepicker .xdsoft_calendar td.xdsoft_disabled,
|
||||
.xdsoft_datetimepicker .xdsoft_time_box >div >div.xdsoft_disabled {
|
||||
opacity: 0.5;
|
||||
-ms-filter: "progid:DXImageTransform.Microsoft.Alpha(Opacity=50)";
|
||||
cursor: default;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_calendar td.xdsoft_other_month.xdsoft_disabled {
|
||||
opacity: 0.2;
|
||||
-ms-filter: "progid:DXImageTransform.Microsoft.Alpha(Opacity=20)";
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_calendar td:hover,
|
||||
.xdsoft_datetimepicker .xdsoft_timepicker .xdsoft_time_box >div >div:hover {
|
||||
color: #fff !important;
|
||||
background: #ff8000 !important;
|
||||
box-shadow: none !important;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_calendar td.xdsoft_current.xdsoft_disabled:hover,
|
||||
.xdsoft_datetimepicker .xdsoft_timepicker .xdsoft_time_box>div>div.xdsoft_current.xdsoft_disabled:hover {
|
||||
background: #33aaff !important;
|
||||
box-shadow: #178fe5 0 1px 3px 0 inset !important;
|
||||
color: #fff !important;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_calendar td.xdsoft_disabled:hover,
|
||||
.xdsoft_datetimepicker .xdsoft_timepicker .xdsoft_time_box >div >div.xdsoft_disabled:hover {
|
||||
color: inherit !important;
|
||||
background: inherit !important;
|
||||
box-shadow: inherit !important;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_calendar th {
|
||||
font-weight: 700;
|
||||
text-align: center;
|
||||
color: #999;
|
||||
cursor: default;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_copyright {
|
||||
color: #ccc !important;
|
||||
font-size: 10px;
|
||||
clear: both;
|
||||
float: none;
|
||||
margin-left: 8px;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker .xdsoft_copyright a { color: #eee !important }
|
||||
.xdsoft_datetimepicker .xdsoft_copyright a:hover { color: #aaa !important }
|
||||
|
||||
.xdsoft_time_box {
|
||||
position: relative;
|
||||
border: 1px solid #ccc;
|
||||
}
|
||||
.xdsoft_scrollbar >.xdsoft_scroller {
|
||||
background: #ccc !important;
|
||||
height: 20px;
|
||||
border-radius: 3px;
|
||||
}
|
||||
.xdsoft_scrollbar {
|
||||
position: absolute;
|
||||
width: 7px;
|
||||
right: 0;
|
||||
top: 0;
|
||||
bottom: 0;
|
||||
cursor: pointer;
|
||||
}
|
||||
.xdsoft_scroller_box {
|
||||
position: relative;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker.xdsoft_dark {
|
||||
box-shadow: 0 5px 15px -5px rgba(255, 255, 255, 0.506);
|
||||
background: #000;
|
||||
border-bottom: 1px solid #444;
|
||||
border-left: 1px solid #333;
|
||||
border-right: 1px solid #333;
|
||||
border-top: 1px solid #333;
|
||||
color: #ccc;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_timepicker .xdsoft_time_box {
|
||||
border-bottom: 1px solid #222;
|
||||
}
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_timepicker .xdsoft_time_box >div >div {
|
||||
background: #0a0a0a;
|
||||
border-top: 1px solid #222;
|
||||
color: #999;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_label {
|
||||
background-color: #000;
|
||||
}
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_label > .xdsoft_select {
|
||||
border: 1px solid #333;
|
||||
background: #000;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_label > .xdsoft_select > div > .xdsoft_option:hover {
|
||||
color: #000;
|
||||
background: #007fff;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_label > .xdsoft_select > div > .xdsoft_option.xdsoft_current {
|
||||
background: #cc5500;
|
||||
box-shadow: #b03e00 0 1px 3px 0 inset;
|
||||
color: #000;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_label i,
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_prev,
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_next,
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_today_button {
|
||||
background-image: url(data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAGQAAAAeCAYAAADaW7vzAAAAGXRFWHRTb2Z0d2FyZQBBZG9iZSBJbWFnZVJlYWR5ccllPAAAAyJpVFh0WE1MOmNvbS5hZG9iZS54bXAAAAAAADw/eHBhY2tldCBiZWdpbj0i77u/IiBpZD0iVzVNME1wQ2VoaUh6cmVTek5UY3prYzlkIj8+IDx4OnhtcG1ldGEgeG1sbnM6eD0iYWRvYmU6bnM6bWV0YS8iIHg6eG1wdGs9IkFkb2JlIFhNUCBDb3JlIDUuMy1jMDExIDY2LjE0NTY2MSwgMjAxMi8wMi8wNi0xNDo1NjoyNyAgICAgICAgIj4gPHJkZjpSREYgeG1sbnM6cmRmPSJodHRwOi8vd3d3LnczLm9yZy8xOTk5LzAyLzIyLXJkZi1zeW50YXgtbnMjIj4gPHJkZjpEZXNjcmlwdGlvbiByZGY6YWJvdXQ9IiIgeG1sbnM6eG1wPSJodHRwOi8vbnMuYWRvYmUuY29tL3hhcC8xLjAvIiB4bWxuczp4bXBNTT0iaHR0cDovL25zLmFkb2JlLmNvbS94YXAvMS4wL21tLyIgeG1sbnM6c3RSZWY9Imh0dHA6Ly9ucy5hZG9iZS5jb20veGFwLzEuMC9zVHlwZS9SZXNvdXJjZVJlZiMiIHhtcDpDcmVhdG9yVG9vbD0iQWRvYmUgUGhvdG9zaG9wIENTNiAoV2luZG93cykiIHhtcE1NOkluc3RhbmNlSUQ9InhtcC5paWQ6QUExQUUzOTA0M0UyMTFFNDlBM0FFQTJENTExRDVBODYiIHhtcE1NOkRvY3VtZW50SUQ9InhtcC5kaWQ6QUExQUUzOTE0M0UyMTFFNDlBM0FFQTJENTExRDVBODYiPiA8eG1wTU06RGVyaXZlZEZyb20gc3RSZWY6aW5zdGFuY2VJRD0ieG1wLmlpZDpBQTFBRTM4RTQzRTIxMUU0OUEzQUVBMkQ1MTFENUE4NiIgc3RSZWY6ZG9jdW1lbnRJRD0ieG1wLmRpZDpBQTFBRTM4RjQzRTIxMUU0OUEzQUVBMkQ1MTFENUE4NiIvPiA8L3JkZjpEZXNjcmlwdGlvbj4gPC9yZGY6UkRGPiA8L3g6eG1wbWV0YT4gPD94cGFja2V0IGVuZD0iciI/Pp0VxGEAAAIASURBVHja7JrNSgMxEMebtgh+3MSLr1T1Xn2CHoSKB08+QmR8Bx9A8e7RixdB9CKCoNdexIugxFlJa7rNZneTbLIpM/CnNLsdMvNjM8l0mRCiQ9Ye61IKCAgZAUnH+mU3MMZaHYChBnJUDzWOFZdVfc5+ZFLbrWDeXPwbxIqrLLfaeS0hEBVGIRQCEiZoHQwtlGSByCCdYBl8g8egTTAWoKQMRBRBcZxYlhzhKegqMOageErsCHVkk3hXIFooDgHB1KkHIHVgzKB4ADJQ/A1jAFmAYhkQqA5TOBtocrKrgXwQA8gcFIuAIO8sQSA7hidvPwaQGZSaAYHOUWJABhWWw2EMIH9QagQERU4SArJXo0ZZL18uvaxejXt/Em8xjVBXmvFr1KVm/AJ10tRe2XnraNqaJvKE3KHuUbfK1E+VHB0q40/y3sdQSxY4FHWeKJCunP8UyDdqJZenT3ntVV5jIYCAh20vT7ioP8tpf6E2lfEMwERe+whV1MHjwZB7PBiCxcGQWwKZKD62lfGNnP/1poFAA60T7rF1UgcKd2id3KDeUS+oLWV8DfWAepOfq00CgQabi9zjcgJVYVD7PVzQUAUGAQkbNJTBICDhgwYTjDYD6XeW08ZKh+A4pYkzenOxXUbvZcWz7E8ykRMnIHGX1XPl+1m2vPYpL+2qdb8CDAARlKFEz/ZVkAAAAABJRU5ErkJggg==);
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_calendar td,
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_calendar th {
|
||||
background: #0a0a0a;
|
||||
border: 1px solid #222;
|
||||
color: #999;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_calendar th {
|
||||
background: #0e0e0e;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_calendar td.xdsoft_today {
|
||||
color: #cc5500;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_calendar td.xdsoft_default,
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_calendar td.xdsoft_current,
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_timepicker .xdsoft_time_box >div >div.xdsoft_current {
|
||||
background: #cc5500;
|
||||
box-shadow: #b03e00 0 1px 3px 0 inset;
|
||||
color: #000;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_calendar td:hover,
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_timepicker .xdsoft_time_box >div >div:hover {
|
||||
color: #000 !important;
|
||||
background: #007fff !important;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_calendar th {
|
||||
color: #666;
|
||||
}
|
||||
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_copyright { color: #333 !important }
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_copyright a { color: #111 !important }
|
||||
.xdsoft_datetimepicker.xdsoft_dark .xdsoft_copyright a:hover { color: #555 !important }
|
||||
|
||||
.xdsoft_dark .xdsoft_time_box {
|
||||
border: 1px solid #333;
|
||||
}
|
||||
|
||||
.xdsoft_dark .xdsoft_scrollbar >.xdsoft_scroller {
|
||||
background: #333 !important;
|
||||
}
|
||||
.xdsoft_datetimepicker .xdsoft_save_selected {
|
||||
display: block;
|
||||
border: 1px solid #dddddd !important;
|
||||
margin-top: 5px;
|
||||
width: 100%;
|
||||
color: #454551;
|
||||
font-size: 13px;
|
||||
}
|
||||
.xdsoft_datetimepicker .blue-gradient-button {
|
||||
font-family: "museo-sans", "Book Antiqua", sans-serif;
|
||||
font-size: 12px;
|
||||
font-weight: 300;
|
||||
color: #82878c;
|
||||
height: 28px;
|
||||
position: relative;
|
||||
padding: 4px 17px 4px 33px;
|
||||
border: 1px solid #d7d8da;
|
||||
background: -moz-linear-gradient(top, #fff 0%, #f4f8fa 73%);
|
||||
/* FF3.6+ */
|
||||
background: -webkit-gradient(linear, left top, left bottom, color-stop(0%, #fff), color-stop(73%, #f4f8fa));
|
||||
/* Chrome,Safari4+ */
|
||||
background: -webkit-linear-gradient(top, #fff 0%, #f4f8fa 73%);
|
||||
/* Chrome10+,Safari5.1+ */
|
||||
background: -o-linear-gradient(top, #fff 0%, #f4f8fa 73%);
|
||||
/* Opera 11.10+ */
|
||||
background: -ms-linear-gradient(top, #fff 0%, #f4f8fa 73%);
|
||||
/* IE10+ */
|
||||
background: linear-gradient(to bottom, #fff 0%, #f4f8fa 73%);
|
||||
/* W3C */
|
||||
filter: progid:DXImageTransform.Microsoft.gradient( startColorstr='#fff', endColorstr='#f4f8fa',GradientType=0 );
|
||||
/* IE6-9 */
|
||||
}
|
||||
.xdsoft_datetimepicker .blue-gradient-button:hover, .xdsoft_datetimepicker .blue-gradient-button:focus, .xdsoft_datetimepicker .blue-gradient-button:hover span, .xdsoft_datetimepicker .blue-gradient-button:focus span {
|
||||
color: #454551;
|
||||
background: -moz-linear-gradient(top, #f4f8fa 0%, #FFF 73%);
|
||||
/* FF3.6+ */
|
||||
background: -webkit-gradient(linear, left top, left bottom, color-stop(0%, #f4f8fa), color-stop(73%, #FFF));
|
||||
/* Chrome,Safari4+ */
|
||||
background: -webkit-linear-gradient(top, #f4f8fa 0%, #FFF 73%);
|
||||
/* Chrome10+,Safari5.1+ */
|
||||
background: -o-linear-gradient(top, #f4f8fa 0%, #FFF 73%);
|
||||
/* Opera 11.10+ */
|
||||
background: -ms-linear-gradient(top, #f4f8fa 0%, #FFF 73%);
|
||||
/* IE10+ */
|
||||
background: linear-gradient(to bottom, #f4f8fa 0%, #FFF 73%);
|
||||
/* W3C */
|
||||
filter: progid:DXImageTransform.Microsoft.gradient( startColorstr='#f4f8fa', endColorstr='#FFF',GradientType=0 );
|
||||
/* IE6-9 */
|
||||
}
|
1933
includes/js/dtp/jquery.datetimepicker.js
Normal file
6
includes/js/dtp/jquery.js
vendored
Normal file
28
includes/js/dtp/package.json
Normal file
@ -0,0 +1,28 @@
|
||||
{
|
||||
"name": "jquery-datetimepicker",
|
||||
"version": "2.4.0",
|
||||
"description": "jQuery Plugin DateTimePicker it is DatePicker and TimePicker in one",
|
||||
"main": "jquery.datetimepicker.js",
|
||||
"scripts": {
|
||||
"test": "echo \"Error: no test specified\" && exit 1"
|
||||
},
|
||||
"repository": {
|
||||
"type": "git",
|
||||
"url": "https://github.com/xdan/datetimepicker.git"
|
||||
},
|
||||
"keywords": [
|
||||
"jquery-plugin",
|
||||
"calendar",
|
||||
"date",
|
||||
"time",
|
||||
"datetime",
|
||||
"datepicker",
|
||||
"timepicker"
|
||||
],
|
||||
"author": "Chupurnov <chupurnov@gmail.com> (http://xdsoft.net/)",
|
||||
"license": "MIT",
|
||||
"bugs": {
|
||||
"url": "https://github.com/xdan/datetimepicker/issues"
|
||||
},
|
||||
"homepage": "https://github.com/xdan/datetimepicker"
|
||||
}
|
BIN
includes/js/dtp/screen/1.png
Normal file
After Width: | Height: | Size: 9.5 KiB |
BIN
includes/js/dtp/screen/2.png
Normal file
After Width: | Height: | Size: 5.0 KiB |
BIN
includes/js/dtp/screen/3.1.png
Normal file
After Width: | Height: | Size: 4.3 KiB |
BIN
includes/js/dtp/screen/3.png
Normal file
After Width: | Height: | Size: 2.4 KiB |
1256
includes/js/jquery.datetimeentry.js
Normal file
344
includes/js/jquery.plugin.js
Normal file
@ -0,0 +1,344 @@
|
||||
/* Simple JavaScript Inheritance
|
||||
* By John Resig http://ejohn.org/
|
||||
* MIT Licensed.
|
||||
*/
|
||||
// Inspired by base2 and Prototype
|
||||
(function(){
|
||||
var initializing = false;
|
||||
|
||||
// The base JQClass implementation (does nothing)
|
||||
window.JQClass = function(){};
|
||||
|
||||
// Collection of derived classes
|
||||
JQClass.classes = {};
|
||||
|
||||
// Create a new JQClass that inherits from this class
|
||||
JQClass.extend = function extender(prop) {
|
||||
var base = this.prototype;
|
||||
|
||||
// Instantiate a base class (but only create the instance,
|
||||
// don't run the init constructor)
|
||||
initializing = true;
|
||||
var prototype = new this();
|
||||
initializing = false;
|
||||
|
||||
// Copy the properties over onto the new prototype
|
||||
for (var name in prop) {
|
||||
// Check if we're overwriting an existing function
|
||||
prototype[name] = typeof prop[name] == 'function' &&
|
||||
typeof base[name] == 'function' ?
|
||||
(function(name, fn){
|
||||
return function() {
|
||||
var __super = this._super;
|
||||
|
||||
// Add a new ._super() method that is the same method
|
||||
// but on the super-class
|
||||
this._super = function(args) {
|
||||
return base[name].apply(this, args || []);
|
||||
};
|
||||
|
||||
var ret = fn.apply(this, arguments);
|
||||
|
||||
// The method only need to be bound temporarily, so we
|
||||
// remove it when we're done executing
|
||||
this._super = __super;
|
||||
|
||||
return ret;
|
||||
};
|
||||
})(name, prop[name]) :
|
||||
prop[name];
|
||||
}
|
||||
|
||||
// The dummy class constructor
|
||||
function JQClass() {
|
||||
// All construction is actually done in the init method
|
||||
if (!initializing && this._init) {
|
||||
this._init.apply(this, arguments);
|
||||
}
|
||||
}
|
||||
|
||||
// Populate our constructed prototype object
|
||||
JQClass.prototype = prototype;
|
||||
|
||||
// Enforce the constructor to be what we expect
|
||||
JQClass.prototype.constructor = JQClass;
|
||||
|
||||
// And make this class extendable
|
||||
JQClass.extend = extender;
|
||||
|
||||
return JQClass;
|
||||
};
|
||||
})();
|
||||
|
||||
(function($) { // Ensure $, encapsulate
|
||||
|
||||
/** Abstract base class for collection plugins v1.0.1.
|
||||
Written by Keith Wood (kbwood{at}iinet.com.au) December 2013.
|
||||
Licensed under the MIT (https://github.com/jquery/jquery/blob/master/MIT-LICENSE.txt) license.
|
||||
@module $.JQPlugin
|
||||
@abstract */
|
||||
JQClass.classes.JQPlugin = JQClass.extend({
|
||||
|
||||
/** Name to identify this plugin.
|
||||
@example name: 'tabs' */
|
||||
name: 'plugin',
|
||||
|
||||
/** Default options for instances of this plugin (default: {}).
|
||||
@example defaultOptions: {
|
||||
selectedClass: 'selected',
|
||||
triggers: 'click'
|
||||
} */
|
||||
defaultOptions: {},
|
||||
|
||||
/** Options dependent on the locale.
|
||||
Indexed by language and (optional) country code, with '' denoting the default language (English/US).
|
||||
@example regionalOptions: {
|
||||
'': {
|
||||
greeting: 'Hi'
|
||||
}
|
||||
} */
|
||||
regionalOptions: {},
|
||||
|
||||
/** Names of getter methods - those that can't be chained (default: []).
|
||||
@example _getters: ['activeTab'] */
|
||||
_getters: [],
|
||||
|
||||
/** Retrieve a marker class for affected elements.
|
||||
@private
|
||||
@return {string} The marker class. */
|
||||
_getMarker: function() {
|
||||
return 'is-' + this.name;
|
||||
},
|
||||
|
||||
/** Initialise the plugin.
|
||||
Create the jQuery bridge - plugin name <code>xyz</code>
|
||||
produces <code>$.xyz</code> and <code>$.fn.xyz</code>. */
|
||||
_init: function() {
|
||||
// Apply default localisations
|
||||
$.extend(this.defaultOptions, (this.regionalOptions && this.regionalOptions['']) || {});
|
||||
// Camel-case the name
|
||||
var jqName = camelCase(this.name);
|
||||
// Expose jQuery singleton manager
|
||||
$[jqName] = this;
|
||||
// Expose jQuery collection plugin
|
||||
$.fn[jqName] = function(options) {
|
||||
var otherArgs = Array.prototype.slice.call(arguments, 1);
|
||||
if ($[jqName]._isNotChained(options, otherArgs)) {
|
||||
return $[jqName][options].apply($[jqName], [this[0]].concat(otherArgs));
|
||||
}
|
||||
return this.each(function() {
|
||||
if (typeof options === 'string') {
|
||||
if (options[0] === '_' || !$[jqName][options]) {
|
||||
throw 'Unknown method: ' + options;
|
||||
}
|
||||
$[jqName][options].apply($[jqName], [this].concat(otherArgs));
|
||||
}
|
||||
else {
|
||||
$[jqName]._attach(this, options);
|
||||
}
|
||||
});
|
||||
};
|
||||
},
|
||||
|
||||
/** Set default values for all subsequent instances.
|
||||
@param options {object} The new default options.
|
||||
@example $.plugin.setDefauls({name: value}) */
|
||||
setDefaults: function(options) {
|
||||
$.extend(this.defaultOptions, options || {});
|
||||
},
|
||||
|
||||
/** Determine whether a method is a getter and doesn't permit chaining.
|
||||
@private
|
||||
@param name {string} The method name.
|
||||
@param otherArgs {any[]} Any other arguments for the method.
|
||||
@return {boolean} True if this method is a getter, false otherwise. */
|
||||
_isNotChained: function(name, otherArgs) {
|
||||
if (name === 'option' && (otherArgs.length === 0 ||
|
||||
(otherArgs.length === 1 && typeof otherArgs[0] === 'string'))) {
|
||||
return true;
|
||||
}
|
||||
return $.inArray(name, this._getters) > -1;
|
||||
},
|
||||
|
||||
/** Initialise an element. Called internally only.
|
||||
Adds an instance object as data named for the plugin.
|
||||
@param elem {Element} The element to enhance.
|
||||
@param options {object} Overriding settings. */
|
||||
_attach: function(elem, options) {
|
||||
elem = $(elem);
|
||||
if (elem.hasClass(this._getMarker())) {
|
||||
return;
|
||||
}
|
||||
elem.addClass(this._getMarker());
|
||||
options = $.extend({}, this.defaultOptions, this._getMetadata(elem), options || {});
|
||||
var inst = $.extend({name: this.name, elem: elem, options: options},
|
||||
this._instSettings(elem, options));
|
||||
elem.data(this.name, inst); // Save instance against element
|
||||
this._postAttach(elem, inst);
|
||||
this.option(elem, options);
|
||||
},
|
||||
|
||||
/** Retrieve additional instance settings.
|
||||
Override this in a sub-class to provide extra settings.
|
||||
@param elem {jQuery} The current jQuery element.
|
||||
@param options {object} The instance options.
|
||||
@return {object} Any extra instance values.
|
||||
@example _instSettings: function(elem, options) {
|
||||
return {nav: elem.find(options.navSelector)};
|
||||
} */
|
||||
_instSettings: function(elem, options) {
|
||||
return {};
|
||||
},
|
||||
|
||||
/** Plugin specific post initialisation.
|
||||
Override this in a sub-class to perform extra activities.
|
||||
@param elem {jQuery} The current jQuery element.
|
||||
@param inst {object} The instance settings.
|
||||
@example _postAttach: function(elem, inst) {
|
||||
elem.on('click.' + this.name, function() {
|
||||
...
|
||||
});
|
||||
} */
|
||||
_postAttach: function(elem, inst) {
|
||||
},
|
||||
|
||||
/** Retrieve metadata configuration from the element.
|
||||
Metadata is specified as an attribute:
|
||||
<code>data-<plugin name>="<setting name>: '<value>', ..."</code>.
|
||||
Dates should be specified as strings in this format: 'new Date(y, m-1, d)'.
|
||||
@private
|
||||
@param elem {jQuery} The source element.
|
||||
@return {object} The inline configuration or {}. */
|
||||
_getMetadata: function(elem) {
|
||||
try {
|
||||
var data = elem.data(this.name.toLowerCase()) || '';
|
||||
data = data.replace(/'/g, '"');
|
||||
data = data.replace(/([a-zA-Z0-9]+):/g, function(match, group, i) {
|
||||
var count = data.substring(0, i).match(/"/g); // Handle embedded ':'
|
||||
return (!count || count.length % 2 === 0 ? '"' + group + '":' : group + ':');
|
||||
});
|
||||
data = $.parseJSON('{' + data + '}');
|
||||
for (var name in data) { // Convert dates
|
||||
var value = data[name];
|
||||
if (typeof value === 'string' && value.match(/^new Date\((.*)\)$/)) {
|
||||
data[name] = eval(value);
|
||||
}
|
||||
}
|
||||
return data;
|
||||
}
|
||||
catch (e) {
|
||||
return {};
|
||||
}
|
||||
},
|
||||
|
||||
/** Retrieve the instance data for element.
|
||||
@param elem {Element} The source element.
|
||||
@return {object} The instance data or {}. */
|
||||
_getInst: function(elem) {
|
||||
return $(elem).data(this.name) || {};
|
||||
},
|
||||
|
||||
/** Retrieve or reconfigure the settings for a plugin.
|
||||
@param elem {Element} The source element.
|
||||
@param name {object|string} The collection of new option values or the name of a single option.
|
||||
@param [value] {any} The value for a single named option.
|
||||
@return {any|object} If retrieving a single value or all options.
|
||||
@example $(selector).plugin('option', 'name', value)
|
||||
$(selector).plugin('option', {name: value, ...})
|
||||
var value = $(selector).plugin('option', 'name')
|
||||
var options = $(selector).plugin('option') */
|
||||
option: function(elem, name, value) {
|
||||
elem = $(elem);
|
||||
var inst = elem.data(this.name);
|
||||
if (!name || (typeof name === 'string' && value == null)) {
|
||||
var options = (inst || {}).options;
|
||||
return (options && name ? options[name] : options);
|
||||
}
|
||||
if (!elem.hasClass(this._getMarker())) {
|
||||
return;
|
||||
}
|
||||
var options = name || {};
|
||||
if (typeof name === 'string') {
|
||||
options = {};
|
||||
options[name] = value;
|
||||
}
|
||||
this._optionsChanged(elem, inst, options);
|
||||
$.extend(inst.options, options);
|
||||
},
|
||||
|
||||
/** Plugin specific options processing.
|
||||
Old value available in <code>inst.options[name]</code>, new value in <code>options[name]</code>.
|
||||
Override this in a sub-class to perform extra activities.
|
||||
@param elem {jQuery} The current jQuery element.
|
||||
@param inst {object} The instance settings.
|
||||
@param options {object} The new options.
|
||||
@example _optionsChanged: function(elem, inst, options) {
|
||||
if (options.name != inst.options.name) {
|
||||
elem.removeClass(inst.options.name).addClass(options.name);
|
||||
}
|
||||
} */
|
||||
_optionsChanged: function(elem, inst, options) {
|
||||
},
|
||||
|
||||
/** Remove all trace of the plugin.
|
||||
Override <code>_preDestroy</code> for plugin-specific processing.
|
||||
@param elem {Element} The source element.
|
||||
@example $(selector).plugin('destroy') */
|
||||
destroy: function(elem) {
|
||||
elem = $(elem);
|
||||
if (!elem.hasClass(this._getMarker())) {
|
||||
return;
|
||||
}
|
||||
this._preDestroy(elem, this._getInst(elem));
|
||||
elem.removeData(this.name).removeClass(this._getMarker());
|
||||
},
|
||||
|
||||
/** Plugin specific pre destruction.
|
||||
Override this in a sub-class to perform extra activities and undo everything that was
|
||||
done in the <code>_postAttach</code> or <code>_optionsChanged</code> functions.
|
||||
@param elem {jQuery} The current jQuery element.
|
||||
@param inst {object} The instance settings.
|
||||
@example _preDestroy: function(elem, inst) {
|
||||
elem.off('.' + this.name);
|
||||
} */
|
||||
_preDestroy: function(elem, inst) {
|
||||
}
|
||||
});
|
||||
|
||||
/** Convert names from hyphenated to camel-case.
|
||||
@private
|
||||
@param value {string} The original hyphenated name.
|
||||
@return {string} The camel-case version. */
|
||||
function camelCase(name) {
|
||||
return name.replace(/-([a-z])/g, function(match, group) {
|
||||
return group.toUpperCase();
|
||||
});
|
||||
}
|
||||
|
||||
/** Expose the plugin base.
|
||||
@namespace "$.JQPlugin" */
|
||||
$.JQPlugin = {
|
||||
|
||||
/** Create a new collection plugin.
|
||||
@memberof "$.JQPlugin"
|
||||
@param [superClass='JQPlugin'] {string} The name of the parent class to inherit from.
|
||||
@param overrides {object} The property/function overrides for the new class.
|
||||
@example $.JQPlugin.createPlugin({
|
||||
name: 'tabs',
|
||||
defaultOptions: {selectedClass: 'selected'},
|
||||
_initSettings: function(elem, options) { return {...}; },
|
||||
_postAttach: function(elem, inst) { ... }
|
||||
}); */
|
||||
createPlugin: function(superClass, overrides) {
|
||||
if (typeof superClass === 'object') {
|
||||
overrides = superClass;
|
||||
superClass = 'JQPlugin';
|
||||
}
|
||||
superClass = camelCase(superClass);
|
||||
var className = camelCase(overrides.name);
|
||||
JQClass.classes[className] = JQClass.classes[superClass].extend(overrides);
|
||||
new JQClass.classes[className]();
|
||||
}
|
||||
};
|
||||
|
||||
})(jQuery);
|
4
includes/js/jquery.plugin.min.js
vendored
Normal file
@ -0,0 +1,4 @@
|
||||
/** Abstract base class for collection plugins v1.0.1.
|
||||
Written by Keith Wood (kbwood{at}iinet.com.au) December 2013.
|
||||
Licensed under the MIT (https://github.com/jquery/jquery/blob/master/MIT-LICENSE.txt) license. */
|
||||
(function(){var j=false;window.JQClass=function(){};JQClass.classes={};JQClass.extend=function extender(f){var g=this.prototype;j=true;var h=new this();j=false;for(var i in f){h[i]=typeof f[i]=='function'&&typeof g[i]=='function'?(function(d,e){return function(){var b=this._super;this._super=function(a){return g[d].apply(this,a||[])};var c=e.apply(this,arguments);this._super=b;return c}})(i,f[i]):f[i]}function JQClass(){if(!j&&this._init){this._init.apply(this,arguments)}}JQClass.prototype=h;JQClass.prototype.constructor=JQClass;JQClass.extend=extender;return JQClass}})();(function($){JQClass.classes.JQPlugin=JQClass.extend({name:'plugin',defaultOptions:{},regionalOptions:{},_getters:[],_getMarker:function(){return'is-'+this.name},_init:function(){$.extend(this.defaultOptions,(this.regionalOptions&&this.regionalOptions[''])||{});var c=camelCase(this.name);$[c]=this;$.fn[c]=function(a){var b=Array.prototype.slice.call(arguments,1);if($[c]._isNotChained(a,b)){return $[c][a].apply($[c],[this[0]].concat(b))}return this.each(function(){if(typeof a==='string'){if(a[0]==='_'||!$[c][a]){throw'Unknown method: '+a;}$[c][a].apply($[c],[this].concat(b))}else{$[c]._attach(this,a)}})}},setDefaults:function(a){$.extend(this.defaultOptions,a||{})},_isNotChained:function(a,b){if(a==='option'&&(b.length===0||(b.length===1&&typeof b[0]==='string'))){return true}return $.inArray(a,this._getters)>-1},_attach:function(a,b){a=$(a);if(a.hasClass(this._getMarker())){return}a.addClass(this._getMarker());b=$.extend({},this.defaultOptions,this._getMetadata(a),b||{});var c=$.extend({name:this.name,elem:a,options:b},this._instSettings(a,b));a.data(this.name,c);this._postAttach(a,c);this.option(a,b)},_instSettings:function(a,b){return{}},_postAttach:function(a,b){},_getMetadata:function(d){try{var f=d.data(this.name.toLowerCase())||'';f=f.replace(/'/g,'"');f=f.replace(/([a-zA-Z0-9]+):/g,function(a,b,i){var c=f.substring(0,i).match(/"/g);return(!c||c.length%2===0?'"'+b+'":':b+':')});f=$.parseJSON('{'+f+'}');for(var g in f){var h=f[g];if(typeof h==='string'&&h.match(/^new Date\((.*)\)$/)){f[g]=eval(h)}}return f}catch(e){return{}}},_getInst:function(a){return $(a).data(this.name)||{}},option:function(a,b,c){a=$(a);var d=a.data(this.name);if(!b||(typeof b==='string'&&c==null)){var e=(d||{}).options;return(e&&b?e[b]:e)}if(!a.hasClass(this._getMarker())){return}var e=b||{};if(typeof b==='string'){e={};e[b]=c}this._optionsChanged(a,d,e);$.extend(d.options,e)},_optionsChanged:function(a,b,c){},destroy:function(a){a=$(a);if(!a.hasClass(this._getMarker())){return}this._preDestroy(a,this._getInst(a));a.removeData(this.name).removeClass(this._getMarker())},_preDestroy:function(a,b){}});function camelCase(c){return c.replace(/-([a-z])/g,function(a,b){return b.toUpperCase()})}$.JQPlugin={createPlugin:function(a,b){if(typeof a==='object'){b=a;a='JQPlugin'}a=camelCase(a);var c=camelCase(b.name);JQClass.classes[c]=JQClass.classes[a].extend(b);new JQClass.classes[c]()}}})(jQuery);
|
1059
includes/js/jquery.timeentry.js
Normal file
6
includes/js/jquery.timeentry.min.js
vendored
Normal file
73
includes/login-post.php
Normal file
@ -0,0 +1,73 @@
|
||||
<?php
|
||||
|
||||
// Initialize session
|
||||
// server should keep session data for AT LEAST 1 hour
|
||||
ini_set('session.gc_maxlifetime', 28800);
|
||||
|
||||
// each client should remember their session id for EXACTLY 1 hour
|
||||
session_set_cookie_params(3600);
|
||||
|
||||
session_start();
|
||||
function authenticate($uname, $password) {
|
||||
// Active Directory server
|
||||
$ldap_host = "ldaps://auth.DOMAIN.com";
|
||||
|
||||
// Active Directory DN
|
||||
$ldap_dn = "cn=users,dc=DOMAIN,dc=com";
|
||||
|
||||
// user group
|
||||
$ldap_user_group = "users";
|
||||
|
||||
// Supervisor group
|
||||
$ldap_super_group = "supervisors";
|
||||
|
||||
// Login, for purposes of constructing $user login
|
||||
$login = "uid=$uname,cn=users,dc=DOMAIN,dc=com";
|
||||
|
||||
// connect to active directory
|
||||
$ldap = ldap_connect($ldap_host);
|
||||
|
||||
// verify user and password
|
||||
if($bind = @ldap_bind($ldap, $login, $password)) {
|
||||
// valid
|
||||
// check presence in groups
|
||||
$filter = "(uid=" . $uname . ")";
|
||||
|
||||
// get list of groups $uname is a member of
|
||||
$attr = array("memberOf");
|
||||
$result = ldap_search($ldap, $ldap_dn, $filter, $attr) or exit("Unable to search LDAP server");
|
||||
$groups = ldap_get_entries($ldap, $result);
|
||||
|
||||
// get use name from ldap
|
||||
$name_attr = array("cn");
|
||||
$name_result = ldap_search($ldap, $ldap_dn, $filter, $name_attr) or exit("Unable to search LDAP server");
|
||||
$name = ldap_get_entries($ldap, $name_result);
|
||||
|
||||
// check groups
|
||||
foreach($groups[0]['memberof'] as $grps) {
|
||||
// is regular user
|
||||
if (strpos($grps, $ldap_user_group)) $access = 1;
|
||||
|
||||
// is supervisor
|
||||
if (strpos($grps, $ldap_super_group)) $access = 1;
|
||||
|
||||
}
|
||||
|
||||
if ($access != 0) {
|
||||
// establish session variables for user, access level, photo and name
|
||||
$_SESSION['uname'] = $uname;
|
||||
$_SESSION['access'] = $access;
|
||||
ldap_unbind($ldap);
|
||||
return true;
|
||||
} else {
|
||||
// user has no rights
|
||||
ldap_unbind($ldap);
|
||||
return false;
|
||||
}
|
||||
|
||||
} else {
|
||||
// invalid name or password
|
||||
return false;
|
||||
}
|
||||
}
|
||||
?>
|
16
includes/menu.php
Normal file
@ -0,0 +1,16 @@
|
||||
<html>
|
||||
<head>
|
||||
<link rel="stylesheet" type="text/css" href="css/menu.css">
|
||||
</head>
|
||||
<body>
|
||||
<nav>
|
||||
<ul>
|
||||
<li><a href="index.php">Home</a></li>
|
||||
<li><a href="addevent.php">Add an Event</a></li>
|
||||
<li><a href="search.php">Search</a></li>
|
||||
<li><a href="http://bms.int.liquidweb.com/" target=blank>BMS.int</a></li>
|
||||
<li><a href="logout.php">Logout</a></li>
|
||||
</ul>
|
||||
</nav>
|
||||
</body>
|
||||
</html>
|
4
includes/select_alert.php
Normal file
@ -0,0 +1,4 @@
|
||||
<?php
|
||||
include "classes/select.class.php";
|
||||
echo $opt->ShowAlerts();
|
||||
?>
|
4
includes/select_dc.php
Normal file
@ -0,0 +1,4 @@
|
||||
<?php
|
||||
include "classes/select.class.php";
|
||||
echo $opt->ShowDCs();
|
||||
?>
|
4
includes/select_unit.php
Normal file
@ -0,0 +1,4 @@
|
||||
<?php
|
||||
include "classes/select.class.php";
|
||||
echo $opt->ShowUnits();
|
||||
?>
|
4
includes/select_utype.php
Normal file
@ -0,0 +1,4 @@
|
||||
<?php
|
||||
include "classes/select.class.php";
|
||||
echo $opt->ShowUnitType();
|
||||
?>
|
23
includes/update_event.php
Normal file
@ -0,0 +1,23 @@
|
||||
<?php
|
||||
include "db_config.php";
|
||||
$conn = mysqli_connect($servername, $username, $password, $db);
|
||||
|
||||
$event_id = mysqli_real_escape_string($conn, $_POST['event']);
|
||||
$description = mysqli_real_escape_string($conn, $_POST['description']);
|
||||
$is_ongoing = mysqli_real_escape_string($conn, $_POST['is_ongoing']);
|
||||
$end_date_time = mysqli_real_escape_string($conn, $_POST['end_date_time']);
|
||||
$user = mysqli_real_escape_string($conn, $_POST['user']);
|
||||
|
||||
//Insert event to events table
|
||||
$event = "UPDATE events SET description='$description', is_ongoing='$is_ongoing', date_time_end='$end_date_time', user='$user' WHERE event_id='$event_id'";
|
||||
|
||||
$result = mysqli_query($conn, $event);
|
||||
if($result){
|
||||
echo("Event updated, redirecting...");
|
||||
sleep (2);
|
||||
header('Location: ../index.php');
|
||||
exit();
|
||||
} else{
|
||||
echo('Error! Please <a href="javascript:history.back()">go back</a> and try again');
|
||||
}
|
||||
?>
|
66
index.php
Normal file
@ -0,0 +1,66 @@
|
||||
<?php
|
||||
//cleanup old sessions
|
||||
gc_collect_cycles();
|
||||
//start php session
|
||||
session_start();
|
||||
//if user not logged in, force to login.php
|
||||
if( $_SESSION['access'] != 1 ) {
|
||||
require( 'login.php' );
|
||||
} else {
|
||||
?>
|
||||
<html>
|
||||
<head>
|
||||
<link rel="stylesheet" type="text/css" href="css/style.css">
|
||||
<link rel="shortcut icon" href="favicon.ico" />
|
||||
<title>BMS Events</title>
|
||||
</head>
|
||||
<body>
|
||||
<div class=header>
|
||||
<h1>Liquidweb BMS Events</h1>
|
||||
<?php
|
||||
if(isset($_SESSION['uname'])) {
|
||||
echo "Hello, ";
|
||||
print_r($_SESSION['uname']);
|
||||
}
|
||||
include("includes/menu.php"); ?>
|
||||
</div>
|
||||
<div class=content>
|
||||
<h3>Current Ongoing Events</h3>
|
||||
<?php include "includes/db_config.php";
|
||||
$conn1 = new mysqli($servername, $username, $password, $db);
|
||||
if ($conn1->connect_error) {
|
||||
die("Connection Failed: " . $conn1->connect_error);
|
||||
}
|
||||
$sql1 = "SELECT * FROM events AS events INNER JOIN units AS units ON events.unit_id=units.unit_id INNER JOIN alerts as alerts ON events.alert_id=alerts.alert_id where is_ongoing=1;";
|
||||
$result1 = $conn1->query($sql1);
|
||||
if ($result1->num_rows >0){
|
||||
echo "<table align='center'><tr><th>Event ID</th><th>Unit</th><th>Alert</th><th>Start Date and Time</th><th>Description</th><th>End Date and Time</th><th>User</th><th>Edit</th></tr>";
|
||||
while ($row1 = $result1->fetch_assoc()) {
|
||||
echo "<tr><td><a href=https://utilities.mon.liquidweb.com/bms/viewevent.php?eventid=".$row1["event_id"]." target=_blank>".$row1["event_id"]."</a></td><td>".$row1["unit_name"]."</td><td>".$row1["alert_name"]."</td><td>".$row1["date_time_start"]."</td><td>".$row1["description"]."</td><td>".$row1["date_time_end"]."</td><td>".$row1["user"]."</td><td><a href=http://utilities.mon.liquidweb.com/bms/editevent.php?event_id=".$row1["event_id"]." target=blank>Edit</a></td></tr> ";
|
||||
}
|
||||
echo "</table>";
|
||||
} else {
|
||||
echo "<h5>No Ongoing Events at This Time</h5>";
|
||||
}
|
||||
if ($conn1->connect_error) {
|
||||
die("Connection Failed: " . $conn2->connect_error);
|
||||
}
|
||||
$sql2 = "SELECT * FROM events AS events INNER JOIN units AS units ON events.unit_id=units.unit_id INNER JOIN alerts as alerts ON events.alert_id=alerts.alert_id where is_ongoing=0 ORDER BY date_time_end DESC LIMIT 10;";
|
||||
$result2 = $conn1->query($sql2);
|
||||
if ($result2->num_rows >0){
|
||||
echo "<h3>Previous 10 Events</h3>";
|
||||
echo "This does not include ongoing events.";
|
||||
echo "<table align='center'><tr><th>Event ID</th><th>Unit</th><th>Alert</th><th>Start Date and Time</th><th>Description</th><th>End Date and Time</th><th>User</th><th>Edit</th></tr>";
|
||||
while ($row2 = $result2->fetch_assoc()) {
|
||||
echo "<tr><td><a href=https://utilities.mon.liquidweb.com/bms/viewevent.php?eventid=".$row2["event_id"]." target=_blank>".$row2["event_id"]."</a></td><td>".$row2["unit_name"]."</td><td>".$row2["alert_name"]."</td><td>".$row2["date_time_start"]."</td><td>".$row2["description"]."</td><td>".$row2["date_time_end"]."</td><td>".$row2["user"]."</td><td><a href=http://utilities.mon.liquidweb.com/bms/editevent.php?event_id=".$row2["event_id"]." target=blank>Edit</a></td></tr> ";
|
||||
}
|
||||
echo "</table>";
|
||||
} else {
|
||||
echo "<h3>Previous 10 Events</h3>";
|
||||
echo "<h5>No Previous Events</h5>";
|
||||
}
|
||||
$conn1->close();
|
||||
?>
|
||||
</body>
|
||||
</html>
|
||||
<?php } ?>
|
79
login.php
Normal file
@ -0,0 +1,79 @@
|
||||
<?php
|
||||
include("includes/login-post.php");
|
||||
|
||||
// check to see if user is logging out
|
||||
if(isset($_GET['out'])) {
|
||||
// destroy session
|
||||
session_unset();
|
||||
$_SESSION = array();
|
||||
unset($_SESSION['uname'],$_SESSION['access']);
|
||||
session_destroy();
|
||||
}
|
||||
|
||||
// check to see if login form has been submitted
|
||||
if(isset($_POST['userLogin'])){
|
||||
// run information through authenticator
|
||||
if(authenticate($_POST['userLogin'],$_POST['userPassword']))
|
||||
{
|
||||
// authentication passed
|
||||
header("Location: index.php");
|
||||
die();
|
||||
} else {
|
||||
// authentication failed
|
||||
$error = 1;
|
||||
}
|
||||
}
|
||||
|
||||
// output error to user
|
||||
if (isset($error)) echo "Login failed: Incorrect user name, password, or rights<br /-->";
|
||||
|
||||
// output logout success
|
||||
if (isset($_GET['out'])) echo "Logout successful";
|
||||
?>
|
||||
<html>
|
||||
<head>
|
||||
<link rel="stylesheet" type="text/css" href="css/style.css">
|
||||
<link rel="shortcut icon" href="favicon.ico" />
|
||||
<title>BMS Event Login</title>
|
||||
</head>
|
||||
<body>
|
||||
<div class=header>
|
||||
<h1>Liquidweb BMS Events</h1>
|
||||
<?php include("includes/menu.php"); ?>
|
||||
</div>
|
||||
<div class=content>
|
||||
<h2>Please Login with LDAP</h2>
|
||||
<form action="login.php" method="post">
|
||||
<table align='center'>
|
||||
<tr>
|
||||
<th colspan='2'>
|
||||
Please Login with LDAP
|
||||
</th>
|
||||
</tr>
|
||||
<tr>
|
||||
<td>
|
||||
Username:
|
||||
</td>
|
||||
<td>
|
||||
<input type="text" name="userLogin" />
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td>
|
||||
Password:
|
||||
</td>
|
||||
<td>
|
||||
<input type="password" name="userPassword" />
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td colspan='2'>
|
||||
<input type="submit" name="submit" value="Login" />
|
||||
</td>
|
||||
</tr>
|
||||
</table>
|
||||
</form>
|
||||
You will be returned to the main BMS status page after login.
|
||||
</div>
|
||||
</body>
|
||||
</html>
|
7
logout.php
Normal file
@ -0,0 +1,7 @@
|
||||
<?php
|
||||
session_start();
|
||||
if(session_destroy()) // Destroying All Sessions
|
||||
{
|
||||
header("Location: index.php"); // Redirecting To Home Page
|
||||
}
|
||||
?>
|
160
search.php
Normal file
@ -0,0 +1,160 @@
|
||||
<?php
|
||||
//start php session
|
||||
session_start();
|
||||
//if user not logged in, force to login.php
|
||||
if( $_SESSION['access'] != 1 ) {
|
||||
require( 'login.php' );
|
||||
} else {
|
||||
|
||||
?>
|
||||
<html>
|
||||
<head>
|
||||
<link rel="stylesheet" type="text/css" href="css/sestyle.css">
|
||||
<link rel="shortcut icon" href="favicon.ico" />
|
||||
<script type="text/javascript" src="includes/jquery-2.1.3.min.js"></script>
|
||||
<link rel="stylesheet" href="//code.jquery.com/ui/1.11.4/themes/smoothness/jquery-ui.css">
|
||||
<link rel="stylesheet" type="text/css" href="includes/js/dtp/jquery.datetimepicker.css"/ >
|
||||
<script src="includes/js/dtp/jquery.js"></script>
|
||||
<script src="includes/js/dtp/jquery.datetimepicker.js"></script>
|
||||
<title>BMS Event Search</title>
|
||||
<script>
|
||||
$(function() {
|
||||
$('#start_date_time').datetimepicker({timepicker:false, format: 'Y/m/d'});
|
||||
$('#end_date_time').datetimepicker({timepicker:false, format: 'Y/m/d'});
|
||||
});
|
||||
</script>
|
||||
|
||||
<script type="text/javascript">
|
||||
$(document).ready(function(){
|
||||
var queryString = $('select_form').serialize();
|
||||
$("select#utype").prop("disabled", true);
|
||||
$("select#unit").prop("disabled", true);
|
||||
$("select#alert").prop("disabled", true);
|
||||
$("select#datacenter").change(function(){
|
||||
$("select#utype").prop("disabled", true);
|
||||
/*$("select#utype").html("<option>wait...</option>");*/
|
||||
var dc_id = $("select#datacenter option:selected").val();
|
||||
$.post("includes/select_utype.php", function(data){
|
||||
$("select#utype").prop("disabled", false);
|
||||
$("select#utype").html(data);
|
||||
});
|
||||
});
|
||||
$("select#utype").change(function(){
|
||||
$("select#unit").prop("disabled", true);
|
||||
/*$("select#unit").html("<option>wait...</option>");*/
|
||||
var utype_id = $("select#utype option:selected").val();
|
||||
$.post("includes/select_unit.php", {utype_id:utype_id, dc_id:$('#datacenter').val()}, function(data){
|
||||
$("select#unit").prop("disabled", false);
|
||||
$("select#unit").html(data);
|
||||
});
|
||||
});
|
||||
// $("select#unit").change(function(){
|
||||
// $("select#alert").prop("disabled", true);
|
||||
// var unit_id = $("select#unit option:selected").val();
|
||||
// $.post("includes/select_alert.php", {utype_id:$('#utype').val()}, function(data){
|
||||
// $("select#alert").prop("disabled", false);
|
||||
// $("select#alert").html(data);
|
||||
// });
|
||||
// });
|
||||
$("form#select_form").submit(function(){
|
||||
var dc = $("select#datacenter option:selected").val();
|
||||
var utype = $("select#utype option:selected").val();
|
||||
if(cat>0 && type>0)
|
||||
{
|
||||
var result = $("select#utype option:selected").html();
|
||||
$("#result").html('your choice: '+result);
|
||||
}
|
||||
else
|
||||
{
|
||||
$("#result").html("you must choose a DC and Unit Type!");
|
||||
}
|
||||
return false;
|
||||
var unit = $("select#unit option:selected").val();
|
||||
if(cat>0 && type>0)
|
||||
{
|
||||
var result = $("select#unit option:selected").html();
|
||||
$("#result").html('your choice: '+result);
|
||||
}
|
||||
else
|
||||
{
|
||||
$("#result").html("you must choose a DC, Unit Type, Alert, and Unit!");
|
||||
}
|
||||
return false;
|
||||
});
|
||||
});
|
||||
</script>
|
||||
</head>
|
||||
<body>
|
||||
<div class=header>
|
||||
<h1>Liquidweb BMS Events</h1>
|
||||
<?php
|
||||
if(isset($_SESSION['uname'])) {
|
||||
echo "Hello, ";
|
||||
print_r($_SESSION['uname']);
|
||||
}
|
||||
echo "<h2>Search</h2>";
|
||||
include("includes/menu.php"); ?>
|
||||
</div>
|
||||
<?php include "includes/classes/select.class.php";?>
|
||||
<br />
|
||||
<form id="select_form" required method="post" action="search_results.php">
|
||||
<table align="center">
|
||||
<tr>
|
||||
<td>
|
||||
Choose a DC:
|
||||
</td>
|
||||
<td>
|
||||
<select name="datacenter" id="datacenter">
|
||||
<?php echo $opt->ShowDCs(); ?>
|
||||
</select>
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td>
|
||||
Choose a Unit Type:
|
||||
</td>
|
||||
<td>
|
||||
<select name="utype" id="utype" >
|
||||
<?php echo $opt->ShowUnitType(); ?>
|
||||
</select>
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td>
|
||||
Choose a unit:
|
||||
</td>
|
||||
<td>
|
||||
<select name="unit" id="unit" >
|
||||
<?php echo $opt->ShowUnits(); ?>
|
||||
</select>
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td>
|
||||
Event Start Date:
|
||||
</td>
|
||||
<td>
|
||||
<input type="text" name="start_date_time" id="start_date_time" />
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td>
|
||||
Event End Date:
|
||||
</td>
|
||||
<td>
|
||||
<input type="text" name="end_date_time" id="end_date_time" />
|
||||
</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td colspan="2" class="ui-helper-center">
|
||||
<input type="submit" value="Search" />
|
||||
</td>
|
||||
</tr>
|
||||
</table>
|
||||
</form>
|
||||
Search by Unit, start date, or end date of the event. The form will accept any or all of these three options.
|
||||
<br />
|
||||
When entering start or end date, enter any portion of the value, and the search should return relevant data.
|
||||
</body>
|
||||
</html>
|
||||
<?php } ?>
|
72
search_results.php
Normal file
@ -0,0 +1,72 @@
|
||||
<?php
|
||||
//start php session
|
||||
session_start();
|
||||
//if user not logged in, force to login.php
|
||||
if( $_SESSION['access'] != 1 ) {
|
||||
require( 'login.php' );
|
||||
} else {
|
||||
include "includes/db_config.php";
|
||||
$conn = mysqli_connect($servername, $username, $password, $db);
|
||||
|
||||
$unit = mysqli_real_escape_string($conn, $_POST['unit']);
|
||||
$start_date_time = mysqli_real_escape_string($conn, $_POST['start_date_time']);
|
||||
$end_date_time = mysqli_real_escape_string($conn, $_POST['end_date_time']);
|
||||
|
||||
|
||||
if(!empty($unit)) {
|
||||
if(!empty($start_date_time)) {
|
||||
if(!empty($end_date_time)) {
|
||||
$query = "SELECT * FROM events AS events INNER JOIN units AS units ON events.unit_id=units.unit_id INNER JOIN alerts as alerts ON events.alert_id=alerts.alert_id WHERE events.unit_id='$unit' AND date_time_start LIKE '%$start_date_time%' AND date_time_end LIKE '%$end_date_time%'";
|
||||
} else {
|
||||
$query = "SELECT * FROM events AS events INNER JOIN units AS units ON events.unit_id=units.unit_id INNER JOIN alerts as alerts ON events.alert_id=alerts.alert_id WHERE events.unit_id='$unit' AND date_time_start LIKE '%$start_date_time%'";
|
||||
}
|
||||
} else {
|
||||
$query = "SELECT * FROM events AS events INNER JOIN units AS units ON events.unit_id=units.unit_id INNER JOIN alerts as alerts ON events.alert_id=alerts.alert_id WHERE events.unit_id='$unit'";
|
||||
}
|
||||
} else {
|
||||
if(!empty($start_date_time)) {
|
||||
if(!empty($end_date_time)) {
|
||||
$query = "SELECT * FROM events AS events INNER JOIN units AS units ON events.unit_id=units.unit_id INNER JOIN alerts as alerts ON events.alert_id=alerts.alert_id WHERE date_time_start LIKE '%$start_date_time%' AND date_time_end LIKE '%$end_date_time%'";
|
||||
} else {
|
||||
$query = "SELECT * FROM events AS events INNER JOIN units AS units ON events.unit_id=units.unit_id INNER JOIN alerts as alerts ON events.alert_id=alerts.alert_id WHERE date_time_start LIKE '%$start_date_time%'";
|
||||
}
|
||||
}
|
||||
}
|
||||
$result = $conn->query($query);
|
||||
if($result->num_rows >0){
|
||||
?>
|
||||
<html>
|
||||
<head>
|
||||
<link rel="stylesheet" type="text/css" href="css/style.css">
|
||||
<link rel="shortcut icon" href="favicon.ico" />
|
||||
<title>BMS Event Search Results</title>
|
||||
</head>
|
||||
<body>
|
||||
<div class=header>
|
||||
<h1>Liquidweb BMS Events</h1>
|
||||
<?php
|
||||
if(isset($_SESSION['uname'])) {
|
||||
echo "Hello, ";
|
||||
print_r($_SESSION['uname']);
|
||||
}
|
||||
echo "<h2>Search Results</h2>";
|
||||
include("includes/menu.php"); ?>
|
||||
</div>
|
||||
<div class=content>
|
||||
<br />
|
||||
<?php
|
||||
if ($result->num_rows >0){
|
||||
echo "<table align='center'><tr><th>Event ID</th><th>Unit</th><th>Alert</th><th>Start Date and Time</th><th>Description</th><th>End Date and Time</th><th>User</th><th>Edit</th></tr>";
|
||||
while ($row1 = $result->fetch_assoc()) {
|
||||
echo "<tr><td><a href=https://utilities.mon.liquidweb.com/bms/viewevent.php?eventid=".$row1["event_id"]." target=_blank>".$row1["event_id"]."</a></td><td>".$row1["unit_name"]."</td><td>".$row1["alert_name"]."</td><td>".$row1["date_time_start"]."</td><td>".$row1["description"]."</td><td>".$row1["date_time_end"]."</td><td>".$row1["user"]."</td><td><a href=http://utilities.mon.liquidweb.com/bms/editevent.php?event_id=".$row1["event_id"]." target=blank>Edit</a></td></tr> ";
|
||||
}
|
||||
echo "</table>";
|
||||
}
|
||||
|
||||
} else{
|
||||
echo('No Results Found! Please <a href="javascript:history.back()">Go back</a> and try again');
|
||||
}
|
||||
?>
|
||||
</body>
|
||||
</html>
|
||||
<?php } ?>
|
70
viewevent.php
Normal file
@ -0,0 +1,70 @@
|
||||
<?php
|
||||
//start php session
|
||||
session_start();
|
||||
//if user not logged in, force to login.php
|
||||
if( $_SESSION['access'] != 1 ) {
|
||||
require( 'login.php' );
|
||||
} else {
|
||||
|
||||
?>
|
||||
<html>
|
||||
<head>
|
||||
<link rel="stylesheet" type="text/css" href="css/style.css">
|
||||
<link rel="shortcut icon" href="favicon.ico" />
|
||||
<title>BMS Events</title>
|
||||
</head>
|
||||
<body>
|
||||
<div class=header>
|
||||
<h1>Liquidweb BMS Events</h1>
|
||||
<?php
|
||||
if(isset($_SESSION['uname'])) {
|
||||
echo "Hello, ";
|
||||
print_r($_SESSION['uname']);
|
||||
}
|
||||
echo "<h2>View Event</h2>";
|
||||
include("includes/menu.php"); ?>
|
||||
</div>
|
||||
<div class=content>
|
||||
<?php include "includes/db_config.php";
|
||||
$eventid=$_GET['eventid'];
|
||||
$conn1 = new mysqli($servername, $username, $password, $db);
|
||||
if ($conn1->connect_error) {
|
||||
die("Connection Failed: " . $conn1->connect_error);
|
||||
}
|
||||
$sql1 = "SELECT * FROM events AS events INNER JOIN units AS units ON events.unit_id=units.unit_id INNER JOIN alerts as alerts ON events.alert_id=alerts.alert_id WHERE events.event_id=".$eventid.";";
|
||||
$result1 = $conn1->query($sql1);
|
||||
if ($result1->num_rows >0){
|
||||
while ($row1 = $result1->fetch_assoc()) {
|
||||
echo "<br />";
|
||||
// echo "Datacenter: ";
|
||||
// $row1['dc_name'];
|
||||
echo "Unit: ";
|
||||
print_r($row1['unit_name']);
|
||||
echo "<br />";
|
||||
echo "Alert: ";
|
||||
print_r($row1['alert_name']);
|
||||
echo "<br />";
|
||||
echo "Start Date and Time: ";
|
||||
print_r($row1['date_time_start']);
|
||||
echo "<br />";
|
||||
echo "Currently Ongoing? ";
|
||||
if($row1['is_ongoing'] ==1) {
|
||||
echo "Yes";
|
||||
} else {
|
||||
echo "No";
|
||||
}
|
||||
echo "<br />";
|
||||
echo "End Date and Time: ";
|
||||
print_r($row1['date_time_end']);
|
||||
}
|
||||
} else {
|
||||
echo "<h4>ERROR Event ID Not Found</h4>";
|
||||
}
|
||||
if ($conn1->connect_error) {
|
||||
die("Connection Failed: " . $conn1->connect_error);
|
||||
}
|
||||
?>
|
||||
</div>
|
||||
</body>
|
||||
</html>
|
||||
<?php } ?>
|